Page 1 of 2 Canvas provides a full transformation facility that allows you to use any co-ordinate system you want to. In this extract from Ian Elliot's book on JavaScript Graphics we look at how to use custom coordinates.
Now available as a paperback or ebook from Amazon.
JavaScript Bitmap Graphics With Canvas
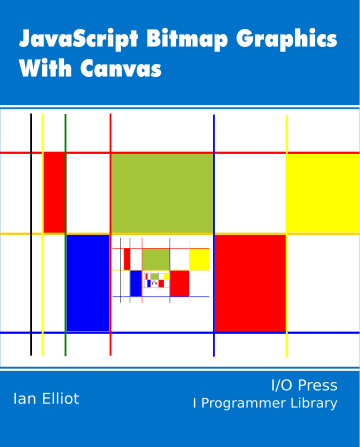
Contents
- JavaScript Graphics
- Getting Started With Canvas
- Drawing Paths
Extract: Basic Paths Extract: SVG Paths Extract: Bezier Curves
- Stroke and Fill
Extract: Stroke Properties Extract: Fill and Holes Extract: Gradient & Pattern Fills
- Transformations
Extract: Transformations Extract: Custom Coordinates Extract Graphics State
- Text
Extract: Text, Typography & SVG Extract: Unicode
- Clipping, Compositing and Effects
Extract: Clipping & Basic Compositing
- Generating Bitmaps
Extract: Introduction To Bitmaps Extract : Animation
- WebWorkers & OffscreenCanvas
Extract: Web Workers Extract: OffscreenCanvas
- Bit Manipulation In JavaScript
Extract: Bit Manipulation
- Typed Arrays
Extract: Typed Arrays
- Files, blobs, URLs & Fetch
Extract: Blobs & Files Extract: Read/Writing Local Files Extract: Fetch API **NEW!
- Image Processing
Extract: ImageData Extract:The Filter API
- 3D WebGL
Extract: WebGL 3D
- 2D WebGL
Extract: WebGL Convolutions
So far we have been working with the default co-ordinate system that, apart from antialiasing concerns, is a pixel co-ordinate system. Canvas provides a full transformation facility that allows you to use any co-ordinate system you want to. Alternatively you can view it as a way of drawing paths at the location and scale that you require.
In Chapter But Not In This Extract
- Transformations
- Transformation Functions
- A Logical Approach to Transforms
Setting Your Own Co-ordinates
When you first use a canvas the co-ordinate system is in terms of the number of pixels in the Bitmap. Often, however, you want to work with a different co‑ordinate system. For example, you might want to work with the origin in the middle and the x and y co-ordinate ranging from -1 to +1.
You can set any co-ordinate system you care to work with using suitable transformations. If your co-ordinate system runs from xmin to xmax and from ymin to ymax you can apply it to the canvas using:
ctx.scale(width/(xmax-xmin),height/(ymax-ymin))
ctx.translate(-xmin,-ymin)
where width and height are the size in pixels of the canvas.
Using this formulation the y co-ordinate increases down the screen, as did the original pixel co-ordinates. If you want the y co-ordinate to increase up the screen then use the transformation:
ctx.scale(width/(xmax-xmin),-height/(ymax-ymin))
ctx.translate(-xmin,-ymax)
and notice the change to ymax in the second line.
So, for example, if you wanted to draw a graph using co-ordinates between 0,0 in the bottom left corner and 10,10 in the top right, i.e. y increasing up the screen, you would use:
var ctx = document.body.appendChild(
createCanvas(500, 500)).getContext("2d");
var xmax = 10;
var xmin = 0;
var ymax = 10;
var ymin = 0;
var width = 500;
var height = 500;
ctx.beginPath();
ctx.scale(width / (xmax - xmin), -height / (ymax - ymin));
ctx.translate(-xmin, -ymax);
ctx.moveTo(0, 0);
ctx.lineTo(0, 10);
ctx.moveTo(0, 0);
ctx.lineTo(10, 0);
ctx.moveTo(0, 0);
ctx.lineTo(10, 10);
ctx.lineWidth = 0.1;
ctx.stroke();
This draws axes and a 45 degree line:
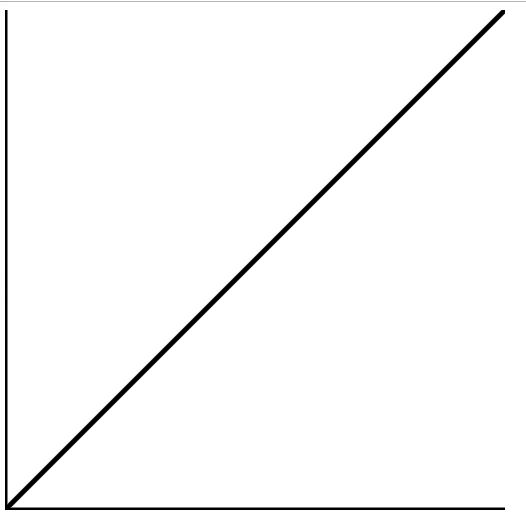
Notice the need to set the lineWidth to take account of the scaling. Also notice that the vertical axis is centered on x=0 and hence half of its thickness is “off” the Canvas. To see this more clearly remove the lineWidth setting.
|