Page 1 of 3 Working with lower-level data is very much part of graphics. This extract from Ian Elliot's book on JavaScript Graphics looks at how to use typed arrays to access graphic data.
Now available as a paperback or ebook from Amazon.
JavaScript Bitmap Graphics With Canvas
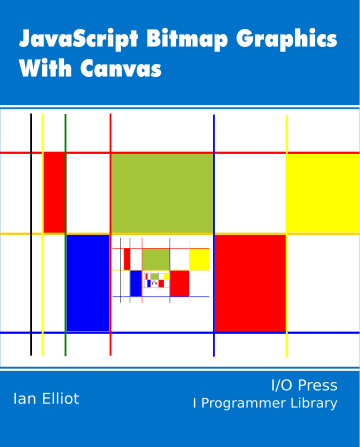
Contents
- JavaScript Graphics
- Getting Started With Canvas
- Drawing Paths
Extract: Basic Paths Extract: SVG Paths Extract: Bezier Curves
- Stroke and Fill
Extract: Stroke Properties Extract: Fill and Holes Extract: Gradient & Pattern Fills
- Transformations
Extract: Transformations Extract: Custom Coordinates Extract Graphics State
- Text
Extract: Text, Typography & SVG Extract: Unicode
- Clipping, Compositing and Effects
Extract: Clipping & Basic Compositing
- Generating Bitmaps
Extract: Introduction To Bitmaps Extract : Animation
- WebWorkers & OffscreenCanvas
Extract: Web Workers Extract: OffscreenCanvas
- Bit Manipulation In JavaScript
Extract: Bit Manipulation
- Typed Arrays
Extract: Typed Arrays
- Files, blobs, URLs & Fetch
Extract: Blobs & Files Extract: Read/Writing Local Files Extract: Fetch API **NEW!
- Image Processing
Extract: ImageData Extract:The Filter API
- 3D WebGL
Extract: WebGL 3D
- 2D WebGL
Extract: WebGL Convolutions
<ASIN:B07XJQDS4Z>
<ASIN:1871962579>
<ASIN:1871962560>
In book but not in this extract:
- The Blob
- The File
- FileReader
- Reading a Local File
- Writing a Local File
Response
There is a new promise-based way to read a file, the Response object, which provides a stream-based way to read data. A stream allows you to read or write chunks of data rather then the whole file at once, which can be useful when the data is being generated continuously or when the file in question is too large to fit into memory. You might consider that reading graphics files is a useful thing to do with large files, but for many graphics formats you can’t process part of a graphics file, so streams are more difficult to use than you might think.
You can construct a Response object using:
var response=new Response(body, init);
where body is one of:
-
Blob
-
BufferSource
-
FormData
-
ReadableStream
-
URLSearchParams
-
USVString
and init sets a range of response properties, which can be ignored in most cases.
Once you have the Response object you can attempt to read the data defined by the body. You can do this as a stream, i.e. read chunks of data as they become ready, or you can use one of the methods that read to completion in a specified format:
-
arrayBuffer()
-
blob()
-
formData()
-
json()
-
text()
None of these formats is particularly useful for a graphics file and it is generally easier to use an Image object to read and decode a file. For an example, let’s implement the reading of a text file from the local file system:
async function displayFile() {
var file = await getFile();
var response= await new Response(file);
var result = await response.text();
console.log(result);
}
Notice that text is always treated as UTF-8 and that ReadFile has an optional parameter that allows you to specify the encoding.
The Response object is a good alternative to using a FileReader simply because it is promise-based, but it is also useful when you really do want to work with a stream. It is also an integral part of the Fetch API.
|