Page 1 of 2 Paths form the outlines that will fill to make graphics. This extract from Ian Elliot's book on JavaScript Graphics looks at how to use SVG paths.
Now available as a paperback or ebook from Amazon.
JavaScript Bitmap Graphics With Canvas
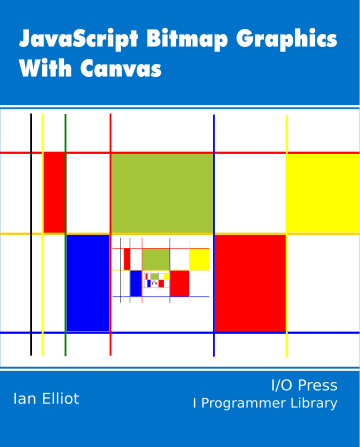
Contents
- JavaScript Graphics
- Getting Started With Canvas
- Drawing Paths
Extract: Basic Paths Extract: SVG Paths Extract: Bezier Curves
- Stroke and Fill
Extract: Stroke Properties Extract: Fill and Holes Extract: Gradient & Pattern Fills
- Transformations
Extract: Transformations Extract: Custom Coordinates Extract Graphics State
- Text
Extract: Text, Typography & SVG Extract: Unicode
- Clipping, Compositing and Effects
Extract: Clipping & Basic Compositing
- Generating Bitmaps
Extract: Introduction To Bitmaps Extract : Animation
- WebWorkers & OffscreenCanvas
Extract: Web Workers Extract: OffscreenCanvas
- Bit Manipulation In JavaScript
Extract: Bit Manipulation
- Typed Arrays
Extract: Typed Arrays
- Files, blobs, URLs & Fetch
Extract: Blobs & Files Extract: Read/Writing Local Files Extract: Fetch API **NEW!
- Image Processing
Extract: ImageData Extract:The Filter API
- 3D WebGL
Extract: WebGL 3D
- 2D WebGL
Extract: WebGL Convolutions
<ASIN:B07XJQDS4Z>
<ASIN:1871962579>
<ASIN:1871962560>
In book but not in this extract
- Paths
- The Default Path Object
- The Rectangle
- Circles and Ellipses
- Ellipse
- The arcTo Function
- Bezier Curves
- Using SVG Paths
You don’t have to know anything about SVG paths to make use of the notation that it introduces to specify a path. The basic idea is that there is a set of single letter commands that you can include in a string and these define the path.
For example, M means moveTo and so a string “M50,50” means the same thing as
moveTo(50,50);
The commands available that are the direct equivalent of function calls are:
-
Mx y = moveTo(x,y)
-
Lx y = lineTo(x,y)
-
Qx1 y1 x y= quadraticCurveTo(x1.y1,x,y)
-
Cx1 y1 x2 y2 x y = bezierCurveTo(x1,y1,x2,y2,x,y)
-
Z = closePath()
You can use a string specifying the path in the Path2D constructor.
For example:
var path=new Path2D(“M50 50 L100 100”);
creates a path that is identical to:
var path=new Path2D();
path.moveTo(50,50);
path.lineTo(100,100);
You might ask at this point what is the advantage? The string notation is much more compact than the function calls. In addition it goes beyond what the function calls can do. For example, if you use the same string commands in lower case then the co-ordinates are all taken to be relative to the current position. This is very useful as to get relative forms of the function calls you have to do arithmetic.
If you change the previous example to:
var path=new Path2D(“m50 50 l100 100”);
it creates a path that moves to cx+50, cy+50 where cx and cy is the current position and then draws a line 100 pixels horizontally and 100 pixels vertically, i.e. to cx+50+100,cy+50+100.
There are also two additional line drawing commands:
which only require you to supply an x or a y co-ordinate. For example “H50” draws a line from the current position to 50,cy.
The commend for drawing an elliptical arc command is A:
This draws an arc, with radii rx and ry, between the current point and x, y. The rot parameter controls the rotation of the radii. The size and sweep flags are confusing at first, but not when you realize that there are usually two ellipses that connect two points – a big arc and a small arc.
Which of these four possible arcs do you want to use to connect the start point to the end point? This is what the size and sweep flags are for. The size flag either selects the small arcs or the large arcs. In this case large or small is measured using the angle specified by sweep. If you set size to 0 then you select the two small arcs:
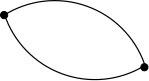
If you set size to 1 you select the two large arcs:
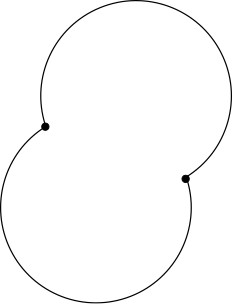
|