Page 1 of 3 Paths are the basic way to create a drawing using Canvas but they can also be used to delimite areas and you can control the way that new graphics are combined with what is already drawn. In this extract from a chapter in my new book on JavaScript Graphics we look at the fundamentals of clipping and compositing.
Now available as a paperback or ebook from Amazon.
JavaScript Bitmap Graphics With Canvas
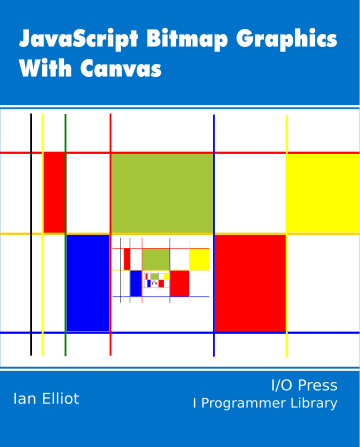
Contents
- JavaScript Graphics
- Getting Started With Canvas
- Drawing Paths
Extract: Basic Paths Extract: SVG Paths Extract: Bezier Curves
- Stroke and Fill
Extract: Stroke Properties Extract: Fill and Holes Extract: Gradient & Pattern Fills
- Transformations
Extract: Transformations Extract: Custom Coordinates Extract Graphics State
- Text
Extract: Text, Typography & SVG Extract: Unicode
- Clipping, Compositing and Effects
Extract: Clipping & Basic Compositing
- Generating Bitmaps
Extract: Introduction To Bitmaps Extract : Animation
- WebWorkers & OffscreenCanvas
Extract: Web Workers Extract: OffscreenCanvas
- Bit Manipulation In JavaScript
Extract: Bit Manipulation
- Typed Arrays
Extract: Typed Arrays **NEW!
- Files, blobs, URLs & Fetch
Extract: Blobs & Files Extract: Read/Writing Local Files
- Image Processing
Extract: ImageData Extract:The Filter API
- 3D WebGL
Extract: WebGL 3D
- 2D WebGL
Extract: WebGL Convolutions
<ASIN:1871962625>
<ASIN:B07XJQDS4Z>
<ASIN:1871962579>
<ASIN:1871962560>
<ASIN:1871962501>
<ASIN:1871962528>
There are ways that you can control how what you draw is combined with what is already drawn on the canvas. You can use a path, not as something to render on the canvas, but as a clipping path which determines what is rendered when you draw other paths. This leads us to consider how what you draw is composited with what is already there. At its very simplest, compositing is just the way color values are logically combined to create new values, but Canvas takes this idea a lot further. Finally there are some special effects which have to be covered somewhere and this is as good a place as any!
Clipping
You have already been relying on clipping without even realizing it. When you set up a canvas and draw on it, anything that goes outside of the canvas rectangle isn't drawn. Notice that while some graphics systems treat drawing outside of the rendering window as an error, Canvas clips the graphic to the rendering window.
You can generalize this idea by defining a clipping path. This can be any path you have created and once the clipping path is set anything you draw is only rendered inside the path.
To set a path as the current clipping path use:
ctx.clip(path,fillrule);
where path is any path and fillrule is optional.
For example,, using the star path introduced earlier we can clip text to its interior:
var myPath = new Path2D();
myPath.moveTo(50, 50);
myPath.lineTo(100, 100);
myPath.lineTo(0, 100);
myPath.lineTo(50, 50);
myPath.moveTo(50, 110);
myPath.lineTo(0, 60);
myPath.lineTo(100, 60);
myPath.lineTo(50, 110);
ctx.stroke(myPath);
ctx.clip(myPath);
ctx.font="normal normal 20px arial";
ctx.fillText("Hello Text World",10,80);
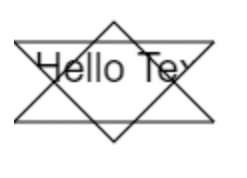
Notice that the path has been rendered before being used as a clipping path so that it can be seen. The text would be clipped as shown, even without rendering the star.
Clipping to the inside of the clipping path depends on what you consider to be "inside" and fillrule works in the same way as described in Chapter 4 in connection with fillStyle. You can set it to "nonzero" which is the default or "evenodd" for the odd-even winding rule. If you change the example to read:
ctx.clip(myPath, "evenodd");
then only the points of the star are considered to be inside.
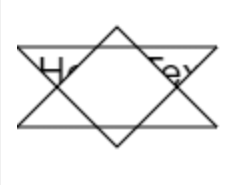
Notice that there is a lot of overlap between fillStyle and clip. You can often produce the same effect as clipping by filling the shape with whatever would be clipped. The exception is for anything that cannot be simply used as a fill. This is why text is used in the example.
Of course, a clipping path doesn't change what has already been drawn and you can change the clipping path as you draw to produce interesting effects. You can only use a real path as a clipping path - shapes you draw directly to the canvas using strokeRect don’t work. You can use the default path and then you simply leave out any parameters to the clip function. Any open paths are automatically closed.
Another interesting problem in using clip is clearing the clip path you have set. If you set a clip path then this is a permanent change to the canvas state. If you use another clip to set another path this is not what happens. Instead, the new clipping region is the intersection of the current clipping area and the new. This means you cannot reset a clipping path by simply specifying a new clipping path.
A the time of writing the only way to rest a clipping path is to use the save method before setting the clipping path and then restore when you want to remove it. Of course, this saves and restores the entire canvas state, but as long as you are aware of it this is generally not a problem. There is a resetClip function in the standard, but it is not well supported.
Compositing
Compositing is the grand terms for how you combine the new pixel value with the existing pixel value. Traditionally compositing rules were simple logical operations, but Canvas has a great many options which attempt to provide additional creative effects. It is difficult to give guidance on how to make use of these. In many cases you simply have to try it out and see if the result is pleasing.
All rendering operations use the same global compositing rule set by:
ctx.globalCompositeOperation=type;
where type is one of the many compositing operations.
Compositing mainly works by using the transparency values of the pixels to control how they are combined, i.e. the Alpha channel is used to determine how pixels from the source and destination are combined. The theory of all this and the names used for compositing operations was worked out in 1984 by Thomas Porter and Tom Duff and presented in their seminal paper titled "Compositing Digital Images". Canvas implements some of the Porter-Duff methods. As these are based on the use of the Alpha channel, they are also known as “alpha compositing modes”.
|