Page 4 of 4
A Simple example
Now we can put this all together and create a program that generates a 25Hz signal on GPIO 2:
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/mcpwm_prelude.h"
void app_main(void)
{
mcpwm_timer_handle_t timer = NULL;
mcpwm_timer_config_t timer_config = {
.group_id = 0,
.clk_src = MCPWM_TIMER_CLK_SRC_DEFAULT,
.resolution_hz = 1000000,
.period_ticks = 20000,
.count_mode = MCPWM_TIMER_COUNT_MODE_UP,
};
mcpwm_new_timer(&timer_config, &timer);
mcpwm_oper_handle_t oper = NULL;
mcpwm_operator_config_t operator_config = {
.group_id = 0,
};
mcpwm_new_operator(&operator_config, &oper);
mcpwm_operator_connect_timer(oper, timer);
mcpwm_gen_handle_t generator = NULL;
mcpwm_generator_config_t generator_config = {
.gen_gpio_num = 2,
};
mcpwm_new_generator(oper, &generator_config, &generator);
mcpwm_gen_timer_event_action_t event_action = {
.direction = MCPWM_TIMER_DIRECTION_UP,
.event = MCPWM_TIMER_EVENT_EMPTY,
.action = MCPWM_GEN_ACTION_TOGGLE
};
mcpwm_generator_set_action_on_timer_event(generator,
MCPWM_GEN_TIMER_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
MCPWM_TIMER_EVENT_FULL, MCPWM_GEN_ACTION_TOGGLE));
mcpwm_timer_enable(timer);
mcpwm_timer_start_stop(timer, MCPWM_TIMER_START_NO_STOP);
}
If you try this program out you will see a 25Hz 50% duty cycle signal.
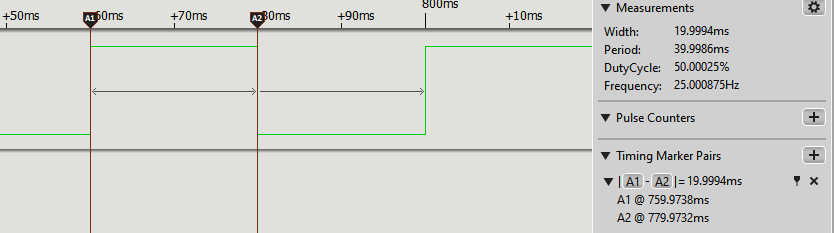
Each edge marks where the timer counter reaches peak value. Thus the timer repeat rate is 50Hz, but the pulse repeat rate is 25Hz.
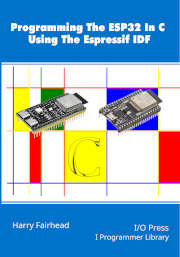
In chapter but not in this extract:
- Using the Comparator
- Duty Cycle
- Two Comparators
- Sync and Phase
- A Complete Program
- Where Next?
Summary
-
The Motor Control PWM MCPWM system is designed to generate PWM signals with complicated phase and duty cycle relationships.
-
There are two MCPWM systems each with six PWM outputs.
-
It includes features designed to be used with motor control – braking, fault handling and speed control.
-
To be as flexible as it needs to be it has a large number of independently programmable components that are initially difficult to master.
-
The key components are the timer, the operator, the comparator and the generator.
-
There are three timers and operators in each MCPWM.
-
The timers are counters that generate events when they are at zero or rollover.
-
The generator changes the PWM signal state depending on events from the timer or comparator.
-
You can generate PWM signals by setting the timer to control the basic frequency, the comparator levels to control the duty cycle.
-
Using two comparators you can generate pulses with a symmetric relationship.
-
You can generate signals with a phase relationship by synchronizing multiple timers with an offset.
-
To make the use of the MCPWM easier it is a good idea to write functions that set up components with reasonable defaults for the application.
By Harry Fairhead
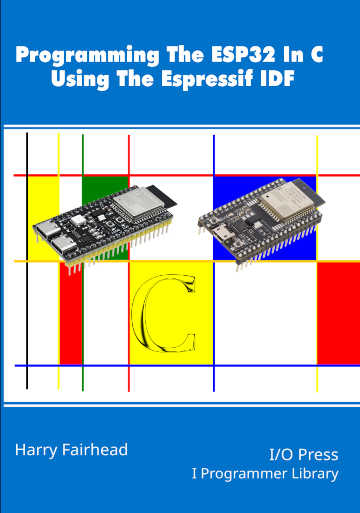
Available as a softback, hardback and kindle from Amazon
Contents
Preface
- The ESP32 – Before We Begin
- Getting Started
- Getting Started With The GPIO
- Simple Output
- Some Electronics
- Simple Input
- Advanced Input – Interrupts
- Pulse Width Modulation
- The Motor Control PWM
Extact: MCPWM First Example ***NEW!
- Controlling Motors And Servos
- Getting Started With The SPI Bus
- Using Analog Sensors
- Using The I2C Bus
- One-Wire Protocols
Extract: The S3's RGB LED
- The Serial Port
- Using WiFi
Extract:Socket Web Client
- Direct To The Hardware
- Free RTOS
<ASIN:1871962919>
<ASIN:187196282X>
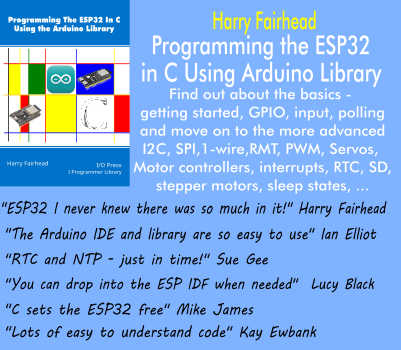
Comments
or email your comment to: comments@i-programmer.info
To be informed about new articles on I Programmer, sign up for our weekly newsletter, subscribe to the RSS feed and follow us on Twitter, Facebook or Linkedin.
|