Page 1 of 3 The ESP32 S3 has WiFi, but getting from a simple connection to a web client is a matter of using sockets. This is an extract from Harry Fairhead's latest book on programming the ESP32 using C and the IDF.
By Harry Fairhead
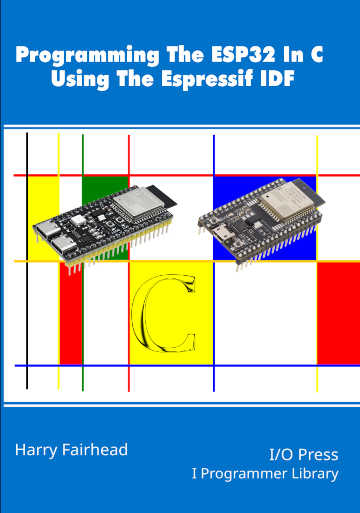
Available as a softback, hardback and kindle from Amazon
Contents
Preface
- The ESP32 – Before We Begin
- Getting Started
- Getting Started With The GPIO
- Simple Output
- Some Electronics
- Simple Input
- Advanced Input – Interrupts
- Pulse Width Modulation
- The Motor Control PWM
Extact: MCPWM First Example ***NEW!
- Controlling Motors And Servos
- Getting Started With The SPI Bus
- Using Analog Sensors
- Using The I2C Bus
- One-Wire Protocols
Extract: The S3's RGB LED
- The Serial Port
- Using WiFi
Extract:Socket Web Client
- Direct To The Hardware
- Free RTOS
<ASIN:1871962919>
<ASIN:187196282X>
The ESP32 comes complete with a radio capable of 2.4GHz WiFi and Bluetooth. Most of the time you can ignore the technical details as there are easy-to-use functions which enable you to connect to a WiFi network and exchange data. In this chapter we look at the basics and how to create and use a WiFi connection. The libraries involved are many and extensive due to the need to cover a wide range of different protocols. A consequence is that there is no way to cover all of them in a reasonable space. This chapter is about getting started and understanding the basic structure of the WiFi and IP infrastructures. When you understand this the rest of the API becomes much easier to understand. The topic of Bluetooth is omitted as it is so varied that it deserves a book to itself.
In book but not in this extract:
- ESP32 Architecture
- The WiFi Stack
- Connecting to WiFi
A Practical Connect Function
Connecting to WiFi is a standard operation and it makes sense to package it in a function. The status of the WiFi connection is communicated to the calling program via a shared static variable.
To make the following examples easier to work with, it is reasonable to put the connect functions into a header file wificonnect.h:
int retry_num = 0;
int wifiStatus = 1000;
static void wifi_event_handler(void* event_handler_arg, esp_event_base_t event_base, int32_t event_id, void* event_data) {
switch (event_id) {
case WIFI_EVENT_STA_START:
wifiStatus = 1001;
break;
case WIFI_EVENT_STA_CONNECTED:
wifiStatus = 1002;
break;
case WIFI_EVENT_STA_DISCONNECTED:
if (retry_num < 5) {
esp_wifi_connect();
retry_num++;
wifiStatus = 1001;
}
break;
case IP_EVENT_STA_GOT_IP:
wifiStatus = 1010;
break;
}
}
void wifiConnect(char* country, char* ssid, char* password) {
nvs_flash_init();
esp_netif_init();
esp_event_loop_create_default();
esp_event_handler_register(WIFI_EVENT, ESP_EVENT_ANY_ID, wifi_event_handler, NULL);
esp_event_handler_register(IP_EVENT, IP_EVENT_STA_GOT_IP, wifi_event_handler, NULL);
wifi_init_config_t wificonfig = WIFI_INIT_CONFIG_DEFAULT();
esp_wifi_init(&wificonfig);
esp_netif_create_default_wifi_sta();
wifi_config_t staconf = {
.sta = {
.threshold.authmode = WIFI_AUTH_WPA_PSK
}
};
strcpy((char*)staconf.sta.ssid, ssid);
strcpy((char*)staconf.sta.password, password);
esp_wifi_set_mode(WIFI_MODE_STA);
esp_wifi_set_config(WIFI_IF_STA, &staconf);
esp_wifi_set_country_code(country, false);
esp_wifi_start();
esp_wifi_connect();
}
|