Page 1 of 3 If you've never encountered WPF (Windows Presentation Foundation) you are missing a versatile tool. This article is part of a series devoted to it. XAML can be confusing - especially if you think it is a markup language like HTML. It isn't. XAML is a general purpose object instantiation language. To find out what this means read on.
The Programmer's Guide To WPF .NET Core
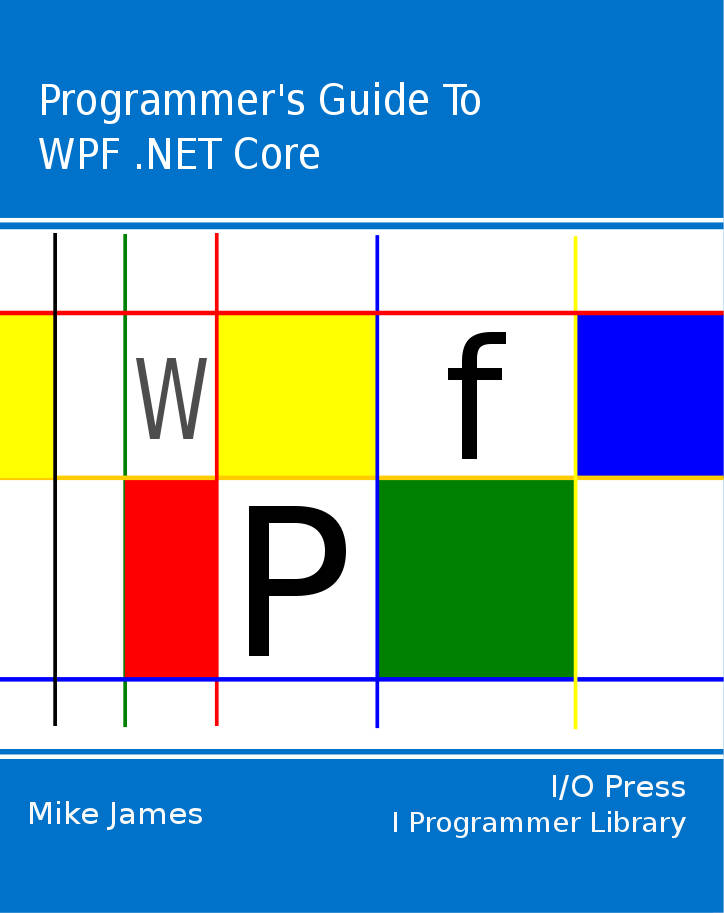
Contents
- Getting Started with WPF
- Getting To Know WPF
- Creating Objects With XAML
- Inside Dependency Properties
- Routed Events ***NEW
- Simple WPF data binding
- FlexGrid - A Lightweight Data Grid
- Using the WPF .NET 4.0 DataGrid
- ISupportInitialize and XAML
- WPF The Easy 3D Way
- BitmapSource: WPF Bitmaps
- Loading Bitmaps: DoEvents and the closure pattern
- Bitmap Effects
- Custom Bitmap Effects - Getting started
- Custom Bitmap Effects - HLSL
- Custom BitmapSource
- Bitmap Coding and Metatdata in WPF
- Custom Shape
- The bitmap transform classes
- Drawing Bitmaps – DrawingImage and DrawingVisual
- RenderTargetBitmap - Visual vector to bitmap
- WriteableBitmap
- BitmapImage and local files
XAML is a, mostly declarative, object instantiation language – that is it’s a way of describing using XML what objects should be created and how they should be initialized before your program starts running.
If you keep this in mind then XAML is becomes a lot more transparent and easier to understand.
Its use in conjunction with WPF is just one of its many possible applications and indeed it has started to appear in other places – Windows Workflow for example and of course it is the UI design language for Windows Store apps i.e. WinRT apps.
To explain exactly what XAML is this article works with custom classes that have nothing to do with WPF so that we can find out about XAML in a completely general context that will help you understand all of its uses potential and current.
Declaritive Instantiation
We could start with a non-WPF project to prove how general XAML is we but this would waste a lot of time adding references and “usings”.
So let’s start with a simple WPF Application, MyProject say, using Visual Studio or your favourite IDE.
You don’t need to modify any of the generated code but you do need to add a simple custom class with which to try out XAML. Right click on the project in the project window and select New,Add, New Item and finally C# class. Call it MyClass:
using System; using System.Collections.Generic; using System.Text;
namespace MyProject { class MyClass { } }
All that is necessary for a class to be instantiable by XAML is that it has a parameterless constructor and it can’t be a nested class.
It can have other constructors but these play no part in its working with XAML. Notice that as structs have a default parameterless constructor provided automatically by the system you can instantiate structs in XAML.
Now that we have our minimal class we can write some XAML to create an instance of it. However first there has to be some way of making the link between the XAML document and the class definition.
This is achieved by importing the class’s namespace into XAML.
You can import the namespace of any assembly and use namespaces to indicate exactly which class you are referring to. In this case we need to import the namespace of the assembly that the XAML file is part of, i.e. the current project.
So moving to the XAML editor we need to add a single line to the <Window> tag:
<Window x:Class="WpfApplication1.Window1" xmlns="http://schemas.microsoft.com/ winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/ winfx/2006/xaml" xmlns:m="clr-namespace:MyProject" Title="Window1" Height="300" Width="300" Loaded="Window_Loaded">
The “clr-namespace” is a special token which is interpreted to mean “get the namespace from the named CLR runtime”.
In general you might also need an “assembly=” token to supply the location of the assembly but in this case it’s assumed to be the current project.
Following this any name prefixed by m: is taken from the namespace of the current project - which is assumed in this case to be WpfApplication1.
The next thing we have to do is to get rid of the <Grid> tags as we cannot nest a general class within a grid – it needs a class that can be displayed.
To create the instance of our class all we have to enter is:
<m:MyClass> </m:MyClass>
between the <Window> and </Window> tag.
Make sure that this is the only code between the windows tags.
The project should now run without errors. If you do see any errors then it will be due to loss of synchronization between namespaces – simply run the project again. The need to keep namespaces and other generated files in sync is one of the problems of splitting instantiation from the runtime.
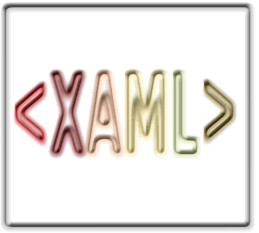
|