Page 1 of 3 One of the great mysteries of WPF is the strangely named "Dependency Properties". In this chapter we learn how dependency properties really work by creating a custom dependency property
The Programmer's Guide To WPF .NET Core
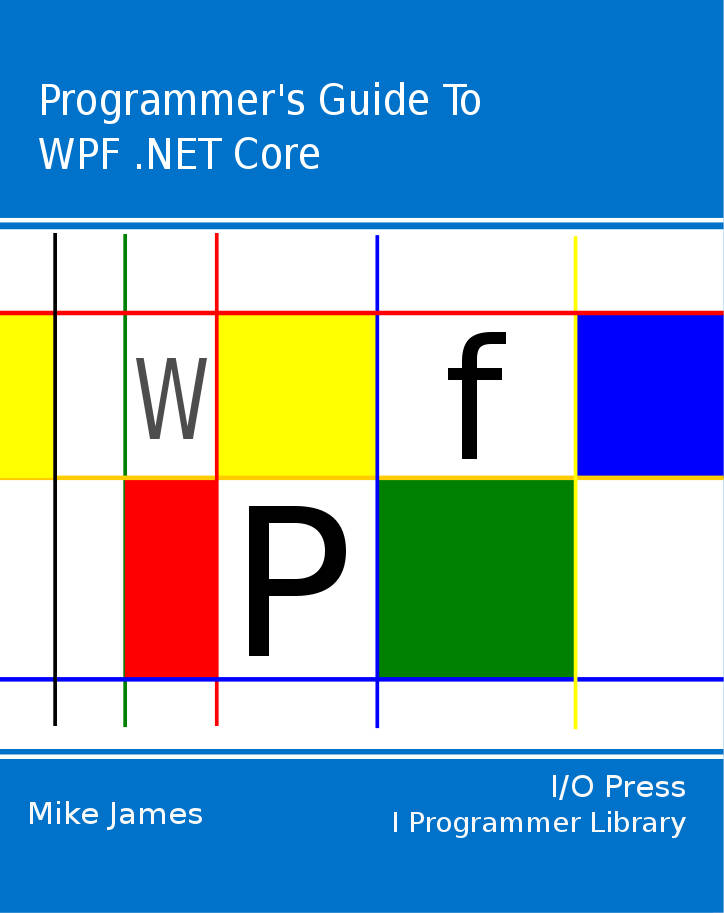
Contents
- Getting Started with WPF
- Getting To Know WPF
- Creating Objects With XAML
- Inside Dependency Properties
- Routed Events ***NEW
- Simple WPF data binding
- FlexGrid - A Lightweight Data Grid
- Using the WPF .NET 4.0 DataGrid
- ISupportInitialize and XAML
- WPF The Easy 3D Way
- BitmapSource: WPF Bitmaps
- Loading Bitmaps: DoEvents and the closure pattern
- Bitmap Effects
- Custom Bitmap Effects - Getting started
- Custom Bitmap Effects - HLSL
- Custom BitmapSource
- Bitmap Coding and Metatdata in WPF
- Custom Shape
- The bitmap transform classes
- Drawing Bitmaps – DrawingImage and DrawingVisual
- RenderTargetBitmap - Visual vector to bitmap
- WriteableBitmap
- BitmapImage and local files
The main reason that they are a mystery is that they are usually explained in very complicated situations with so much extra going on that it can be difficult to work out what is special about dependency properties and what belongs to some other part of WPF.
So let's see if we can simplify it without losing the essential nature of the idea.
CLR Properties
Before going into dependency properties it's worth a quick example of how standard, or CLR (Common Language Runtime), properties work because they play an important role in using dependency properties.
In the days before dependency properties CLR properties were the only sort of properties available but over time the facilities available to implement them have been extended to make things easier.
If you want to define a CLR property then the simplest way is to use the property get set accessor declaration.
For example, to add a property called MyProp of type double to a class you would use:
public class MyClass { private double _MyProp = 0; public double MyProp { get { return _MyProp; } set { _MyProp = value; } } }
The set is called when the property is assigned to and the get is called when it is accessed.
Notice the use of the private _MyProp to store the value of the property between uses - this is referred to as "backing" the property with a private variable.
You can also, and often would, use multiple instructions within the get and set accessors to validate or transform the property value - this is the whole point of using a property rather than just exposing a public variable as a field.
Notice also that there are other ways of backing the property, you could store the value in a file or create it using a random number generator. As long as you come up with a suitable way of implementing a get and set function then you can do what ever you want.
Of course the get and set have to work in a way that is compatible with assignment and the set has to accept a parameter called value and the get has to return a result of the correct type.
Object properties
The key idea behind dependency properties is continuing the upgrade of the idea of a property from a field to a get/set method based property to an object based property.
The CLR property replaces a simple public field by a pair of methods - the set and the get. The next logical step is to create object based properties. That is create a class called ObjProp say and give this the necessary methods and get/set assignment semantics to work as a property.
In this case you could create a property something like:
ObjProp MyProp=new ObjProp(type);
where the type parameter specified the type of the property.
Following this line of reasoning you could now create a framework that supported the way the ObjProp objects behaved. For example, assigning to an ObjProp would store the value of the property and so on. In addition, because each property was an object you could provide sophisticated facilities such as reacting to events on other objects and being able to generate events when the property changes.
Making properties object-based is a good idea but it isn't something to be undertaken lightly - it’s a big job - but the good news is you don't have to because this dependency property do exactly this.
That is, WPF's dependency properties are object-based properties that have lots of additional features and behaviors over and above basic CLR properties.
|