Page 1 of 3 Ajax is the technology that turned the web page into the web app. Since Ajax was invented there have been lots of innovations and frameworks that make web apps easier but a mastery of basic Ajax technique is still important and jQuery makes it easy and cross browser.
Just jQuery Events, Async & AJAX
Is now available as a print book: Amazon
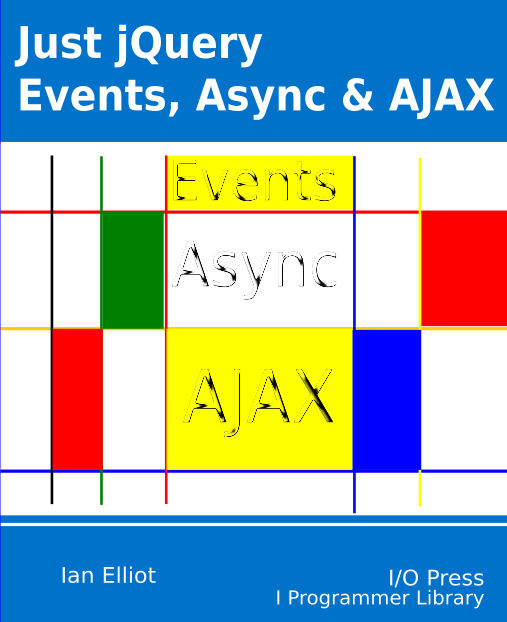
Contents
- Events, Async & Ajax (Book Only)
- Reinventing Events
- Working With Events
- Asynchronous Code
- Consuming Promises
- Using Promises
- WebWorkers
- Ajax the Basics - get
- Ajax the Basics - post
- Ajax - Advanced Ajax To The Server
- Ajax - Advanced Ajax To The Client
- Ajax - Advanced Ajax Transports And JSONP
- Ajax - Advanced Ajax The jsXHR Object
- Ajax - Advanced Ajax Character Coding And Encoding
Also Available:
buy from Amazon
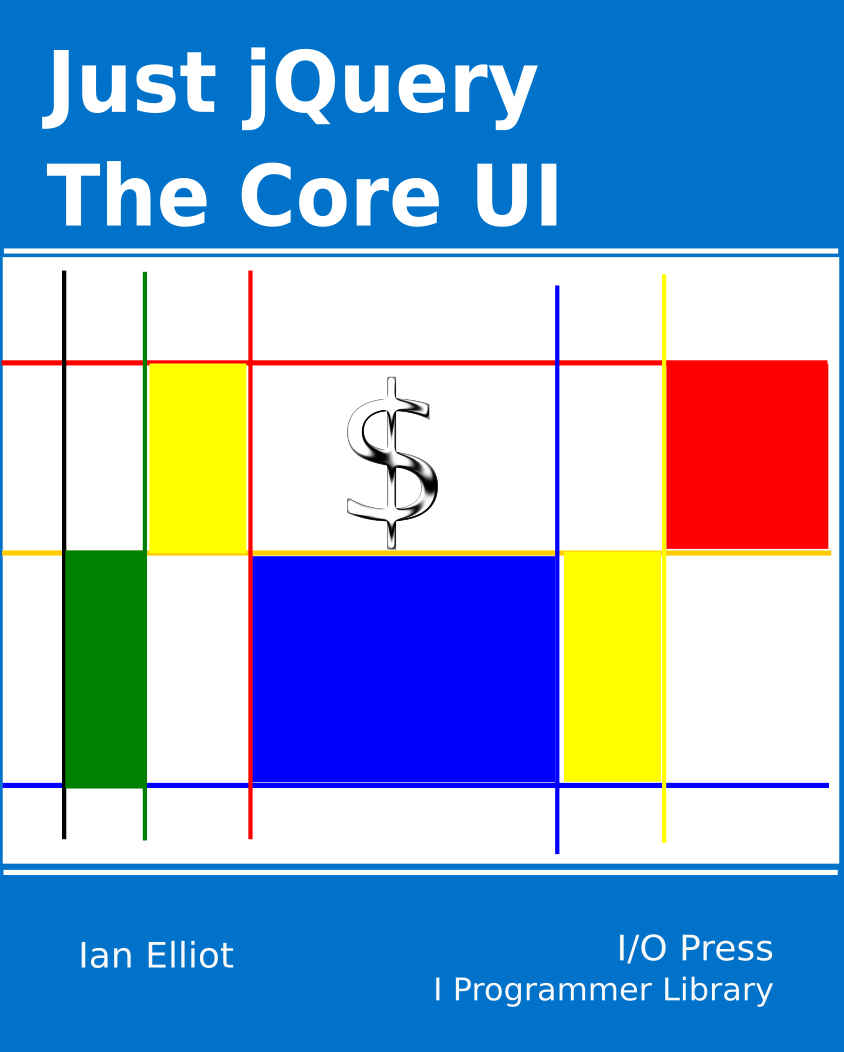
Advanced Attributes
Wanting to make use of Ajax is one of the prime reasons for adopting jQuery. Working with Ajax raw in the browser isn't very difficult but exactly how it works varies according to the browser and jQuery does a very good job of covering up these differences. It isn't difficult to write JavaScript code that works in a wide range of browsers without jQuery but why bother when you get so much extra with jQuery.
First we have to deal with what exactly Ajax is all about and how we might use it.
Before Ajax, a web page loaded and then it loaded all of the resources, image files, JavaScript files and so on that it used. After the initial load there was no way a JavaScript program could download more data until the page was replaced by a new page. The web worked entirely in terms of whole pages and new behaviour or new data from the server was download a whole page at a time.
The Ajax idea is to allow JavaScript to upload and download data to the server under program control. Web pages have always been able to upload data to the server using the HTML form mechanism but this has a limited but very useful applicability.
With Ajax you can, for example, download data from the server to a string say and then use it for what ever purpose you like. Often the new data is HTML which you can then use with jQuery to modify the DOM. In other words the page can be modified without the need to reload the entire page. This gives rise to the idea of the single page web app. A single HTML page can use JavaScript to update itself without ever having to load another page.
If you are planning to write a single page web app then there are frameworks that will make your task easier. You could do the whole job using nothing but jQuery and JavaScript but it would be a lot of work and you would have to reinvent the wheel many times.
jQuery Ajax isn't sufficient to build a complex one page web app. It is incredibly useful when you just need to augment an existing web page to have additional dynamic content.
Similarly you can get by using the standard HTML form to deliver data to the server in many cases. However some times it is just more direct to handle the transfer of data yourself. The main reason for this is when the data doesn't originate in a simple user form.
The bottom line is that jQuery makes Ajax as easy as it can be for small tasks but for anything like a single page application or an MVC style website you need to look for and use a ready build framework.
In this chapter we start off by looking at the basic architecture of an HTTP request and introduce the simplest of jQuery's Ajax method - get.
Starting off with get means that we can avoid dealing with the complications of implementing a program on the server side. Our examples can simply retrieve a file stored on the server. For more complicated situations see the next chapter.
Ajax From The Server's Point Of View
An HTML server only responds to a small number of request types. The only two transactions that are of any interest to Ajax are the Get and Post.
Get is the standard way a web page and other resources are delivered to a client browser. Without going into details what happens is that the client puts together a get request with the URL of the resource it wants and the server finds the file corresponding to the path in the URL.
For example if the client does a get
http://myserver.com/myPage.html
then the server retrieves myPage.html from the local file system from the folder that is the root of the website and sends it to the client.
It really is that simple.
The Post is a little more tricky. When the client sends a Post request its sends a URL to the server and data consisting of name value pairs in the body of the HTTP message. In this case the web server isn't expected to return the web page corresponding to URL to the user but to use the web page to process the data. In fact the web page is returned to the client but if this is all that happened the data passed to the server would be ignored.
For example a Post to
http://myserver.com/myPage.html
does exactly the same as a Get to the same URL, i.e. it returns myPage.html to the client but a payload of data was delivered, and in this case, ignored by the server.
To be of any use the URL specified in a Post has to correspond to some sort of program that can process the data and optionally send a response back to the client. For example the URL often corresponds to a php web page which can access the data the server has received and send some HTML back to the client.
That is the URL in a Post usually specifies a program that will accept the data from the client and send back a response.
Note: if you are working with Netbeans and are trying things out with the embedded server it is important to know that its implementation of Post doesn't return a static file specified in the URL. However it does work if you use the PHP embedded server.
To summarize:
- Get - the server returns the specified file.
- Post - the server receives the specified data in the body of the HTTP request amd returns the specified file.
Notice that it is also possible to pass the server data as part of the URL sent to the server - usually by using the query string. This works but it is limited in the amount of data that can be sent by the maximum length of a URL. For the moment we will ignore the query string approach to sending data to the server - see the next chapter for an example.
jQuery Get
jQuery has an extensive and sophisticated set of Ajax methods but in many cases the "shortcut" methods are all you need as these perform the most common operations. However this said there are often cases were you need the full Ajax method but let us start simple.
The most basic get method is:
$.get(url,function)
where the url is the resource you want to get and the function is the callback that is run if the request is successful.
For example to get the contents of a file called "mydata.txt" stored in the same directory as the current page you would use:
$.get("mydata.txt", function (data) { alert(data); });
The data contained in the file is passed to the callback function in the parameter - data in this case. When the Ajax call is complete the callback displays the data.
We have a lot to say about the data and the way it can be generated by the server but for the moment let's concentrate the basics.
It turns out that including a callback as a parameter is the "old" way of doing things. Modern JavaScript and modern jQuery prefer to use Promises.
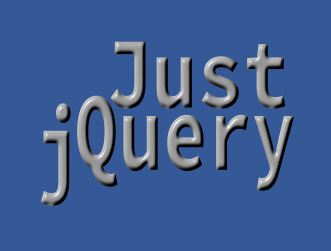
|