Page 1 of 3 The String is the most basic and most useful of the native JavaScript data structures. It can also be used as a starting point for, or be incorporated into, many other data structures. Let's see how it all works.
JavaScript Data Structures
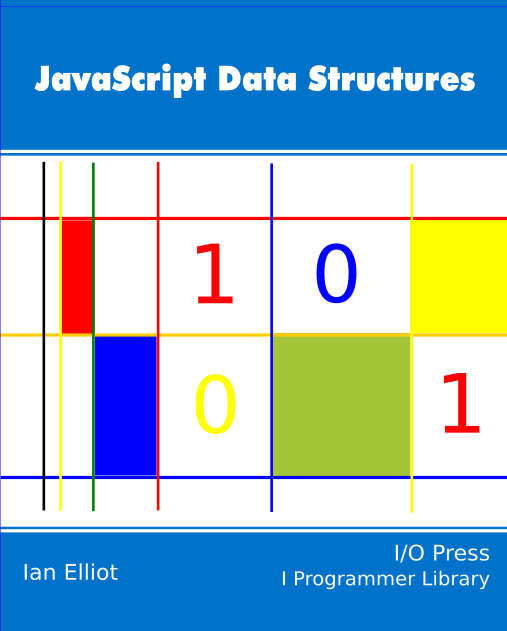
Contents
- The Associative Array
- The String Object
- The Array object
- Speed dating - the art of the JavaScript Date object
- Doing JavaScript Date Calculations
- A Time Interval Object
- Collection Object
- Stacks, Queue & Deque
- The Linked List
- A Lisp-like list
- The Binary Tree
- Bit manipulation
- Typed Arrays I
- Typed Arrays II
- Master JavaScript Regular Expressions
* First Draft
String Object And Literal
You can create a string literal using either " or ' and there is no difference between the two delimiters. That is
"hello string"
and
'hello string'
are treated identically.
The only reason for allowing two different delimiters is that it allows you to write things like:
"The String's Color"
or
'I can "quote" something'
There are some practical things to consider like how do you enter a long string? The most common idiom is to use +=
text="this is the start of a very long string"; text+="and it just goes on"; text+="and on and on...";
You can also use the backslash escape character but this, for reasons that aren't clear, far less common:
text="this is the start of a very long string \ and it just goes on \ and on and on...";
Notice that the backslash has to be the last character on the line.
New in ES2015 is the ability to create string literals that have multiple lines and variables embedded in them i.e. a template literal. A template literal has to be between back ticks and not single or double quotes. To include a variable just include it between curly brackets and a leading dollar sign.
For example:
planet="world"; greeting=`Hello ${planet}'; print greeting;
prints
Hello World
To create a String object you use new in the usual way:
var s=new String("hello string");
You can leave the new out if you want to, but if you do then you don't create a String object but a string literal.
JavaScript takes a very pragmatic approach to strings and objects.
It allows you to define string literals, which are stored as simple character data, and it has a String object, which is less efficient but has all of the methods and properties that you need to make strings useful.
What this means is that when you write:
var s1 = "hello string";
you create a string literal, which is simply a sequence of character codes stored in memory, but when you write:
var s2 = new String("hello string");
you create a string object complete with properties and methods. (Note: the new is essential as without it you just create a string literal.)
This might sound complicated but you don't really have to worry about it too much. The reason is that any time you make use of a string property or method on a string literal JavaScript "wraps" or "boxes" the literal in a String object. Because of this there is very little difference in practice between a string literal and a String Object, even in terms of efficiency.
For example, the length property gives the number of characters in the string and you can use it on a literal or on a String object in exactly the same way:
s1.length s2.length
or even
"hello string".length
There are two fairly obvious and well known places where the difference between a string literal and a string object makes a difference.
The first is in equality testing. If you test for equality between two string literals then it all works as you might expect. However, if you treat String objects as if they were string literals then things seem to go wrong.
For example if you compare two seemingly identical strings:
var s1 = "1+2"; var s2 = new String("1+2");
then s1==s2 is true but s1===s2 is false. Strict equality demands that s1 and s2 are the same object and in this case they are not. What might seem worse is that two String objects with the same value are not considered equal. That is if you define:
var s2 = new String("1+2"); var s3 = new String("1+2");
Then s2==s3 and s2==s3 are both false.
The second place the difference matters is when you use eval to work out the value of a string as an expression or even as a JavaScript program. Eval only evaluates a string literal not a String object. In the case of an object it returns the object's valueOf method. For example:
var s1 = "1+2"; var s2 = new String("1+2");
then eval(s1) returns 3 but eval(s2) returns the string "1+2".
There may be other differences but if so they are less obvious.
All of this sounds as if strings could be a real problem in JavaScript, but in practice most strings are derived from string literals and the use of the String object is rare.
If you want to make sure that string comparisons and eval work in the same way with literals and objects then simply always use valueOf. For example:
s1.valueOf() === s2.valueOf(); s1.valueOf() == s2.valueOf();
are both true, even though s1 is a literal and s2 an object. Similarly:
eval(s1.valueOf()); eval(s2.valueOf());
are both fully evaluated to 3.
<ASIN:1871962579>
<ASIN:1871962560>
|