Page 1 of 4 JavaScript should not be judged as if it was a poor version of the other popular languages - it isn't a Java or a C++ clone. It does things its own way. In particular, it doesn't do inheritance in the same way.
This is an extract from JavaScript Jems: The Amazing Parts.
Jem 2
The Inheritance Tax
“What is the object-oriented way of getting rich? — Inheritance.”
Anonymous
Now available as a book from your local Amazon.
JavaScript Jems: The Amazing Parts
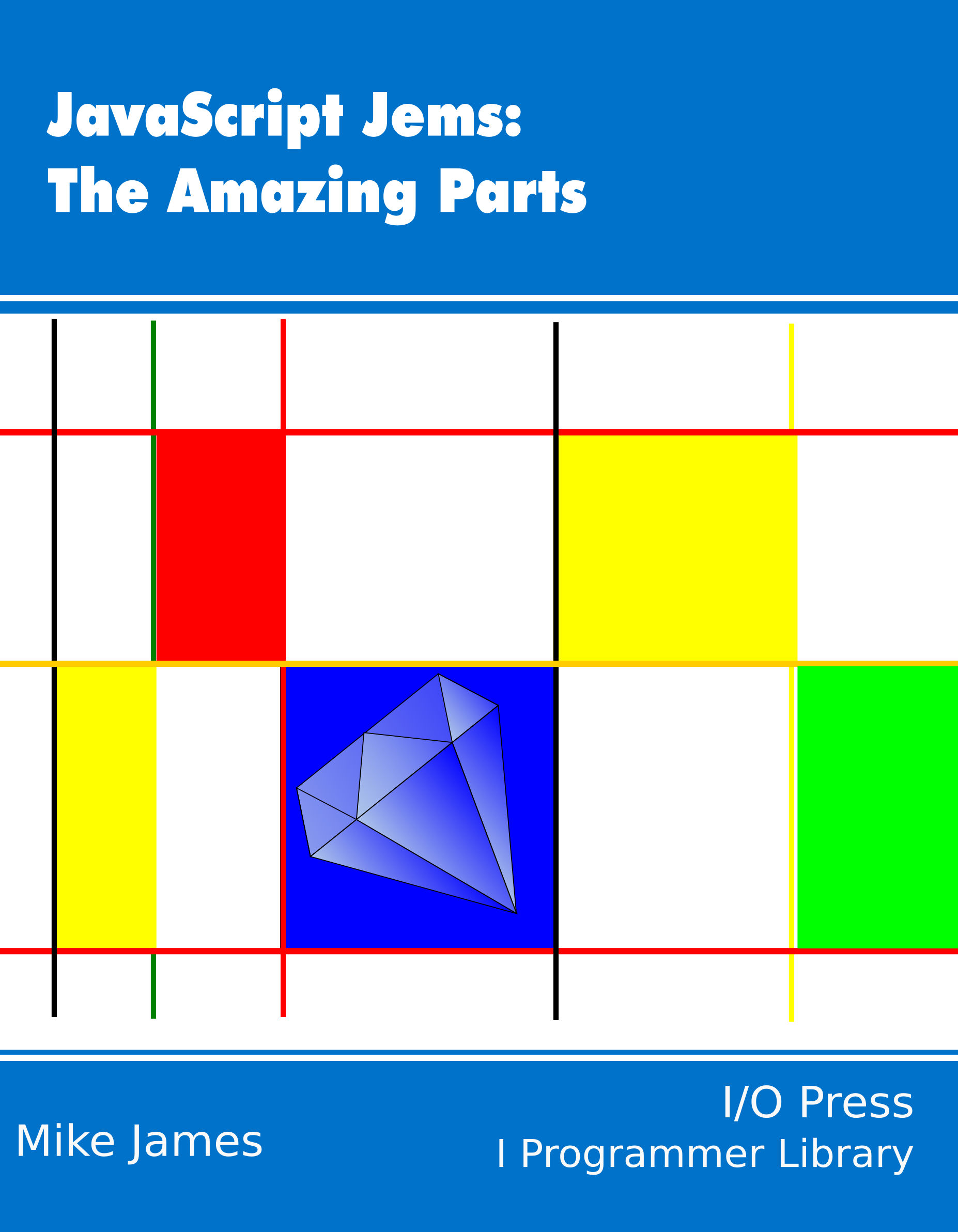
Contents
<ASIN:1871962579>
<ASIN:1871962560>
<ASIN:1871962501>
<ASIN:1871962528>
Another aspect of the class mechanism is inheritance. You can use a class as the basis of another class. The new class has all of the properties and methods of the original class, it inherits from the original class, you can extend the new class by adding new methods and properties, and you can redefine inherited methods if they don't do the job. Before inheritance the common way to reuse code was to use copy-and-paste to duplicate code. Inheritance is dynamic in that if you change the original class the change is propagated to all of the classes that inherit from it. This used to be thought of as the best part of inheritance, but today things aren't so clear cut.
As JavaScript isn't strongly-typed, and it doesn't really do inheritance, some of the examples in this chapter are taken from Java. This is the only way to demonstrate what JavaScript lacks, or should I say avoids, by not supporting inheritance and typing. You can regard this as a Jem of omission. It is worth saying again that this is a contentious issue. Programmers familiar with other languages consider the idea that class, type and inheritance can be dispensed with as ridiculous. So much so that syntax that makes it looks as if JavaScript does support inheritance has been added to ES2015 and there are language "wrappers" like TypeScript that support typing.
Class As Type
In most class-based languages, classes are considered to be types and not objects. The fundamental purpose of the class mechanism is to create a "cookie cutter" template that can be used to stamp out as many instances of the class as required. However, as every instance produced by the class is the same, it leads on to the idea that class defines type.
In a class-based language declaring a class also creates a new data type. That is, in Java:
Class MyClassA(){
lots of properties
}
not only creates a new class, it also adds the new data type, MyClassA, to the type system.
In this way of doing things objects are of a particular type, and variables have to be declared as a particular type, and a variable can only reference objects of that type. Now when you declare a variable of the type:
MyClassA myVariable;
the system knows what myVariable is referring to. This allows the system to check that when you write:
myVariable.myProperty
that myProperty is indeed a property defined on the type. If it isn't then you get a compile time error, which you can correct before it throws a runtime error.
Contrast this with JavaScript, or any untyped language, where myVariable can reference any object and hence you cannot deduce that:
myVariable.myProperty
is valid simply by reading it. You can usually deduce its validity by reading the rest of the program, however. Strong typing makes this aspect of static analysis easier, but this comes at a cost. Given that type occurs in two ways – a variable has a declared type and an object is of a particular type - at its simplest strong typing simply enforces the rule that a variable can only reference an object of its declared type. That is, an instance of a class has a type and only a variable of the same type can reference it.
JavaScript has neither of these two complications - variables are untyped and can reference anything and objects are just objects and there is no type information associated with them. This is a great simplification and should be considered a jem.
|