Page 1 of 4 JavaScript should not be judged as if it was a poor version of the other popular languages - it isn't a Java or a C++ clone. It does things its own way. In particular, every object can be regarded as an anonymous singleton. This is an extract from JavaScript Jems: The Amazing Parts.
Jem 5
Every Object Is An Anonymous Singleton
“We are Anonymous. We are Legion.” The activist group, Anonymous
Now available as a book from your local Amazon.
JavaScript Jems: The Amazing Parts
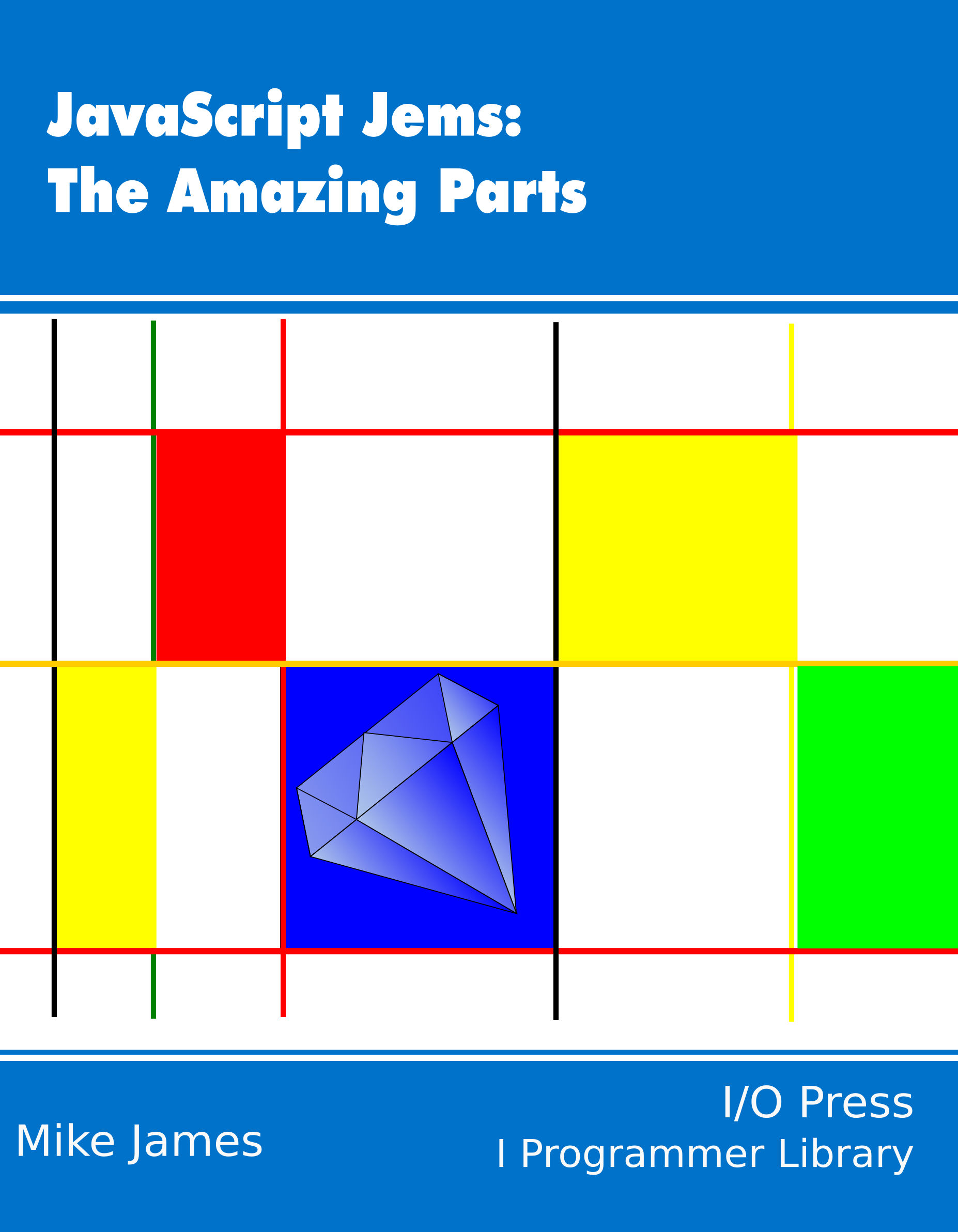
Contents
<ASIN:1871962579>
<ASIN:1871962560>
<ASIN:1871962501>
<ASIN:1871962528>
There are many things that are difficult to unlearn when you start using JavaScript, but perhaps the hardest is the belief that objects are associated with an immutable name and that objects are related to one another in strange and perhaps complex ways. As Jem 1 explains, JavaScript doesn't have class, no matter how hard you try to force the mechanism on it. To really master JavaScript you have to change your world view so that you regard every object as an anonymous singleton. What exactly does this mean? Let’s start with the way objects are referenced.
References
Most programmers, in most languages, treat objects as if they have permanent names, but mostly they don't. The reason is that what we often think of as the object's name is just a reference to it. In the early days of programming a variable was something that held a value - a number, say. Today variables more commonly are used to store a reference to an object. The best way to think of this is as the object being stored in memory separate from the storage allocated to the variable and the variable "points at" or references the object.
That is, when you write:
let myObject = Object.create(
{
myMethod: function Hello() {
alert(this.myProperty2);
},
{
myProperty1: {value: 42},
myProperty2: {value: "deep thought"}
}
);
or create an object in some other way, what happens is that memory is allocated to store the methods and properties of the object created and memory is allocated to store the variable myObject. The variable doesn't store the object itself, only a reference to the object, which takes a lot less space.
Notice that the object doesn't have a name. You access it using the reference stored in myObject, a variable which does have a name.
In other languages a reference would be called a pointer and accessing the object that was pointed at is called dereferencing. In JavaScript, and many other languages, we just take this for granted. The use of references with objects also leads to a distinction between value and reference semantics – although this is unnecessary in a language like JavaScript.
Value semantics is what happens when variables behave as if they store a value, i.e. the “object” is stored “in” the variable. For example, value semantics are often associated with numeric values:
let myVar1=42;
let myVar2=myVar1;
myVar2=0;
In this case the value 42 is stored in myVar1 and then copied into myVar2. When myVar2 is zeroed the value in myVar1 is unchanged, i.e. still 42. In other words, the value assignment makes a copy of the object being stored in the new variable.
Now compare this to reference semantics:
let myVar1={myProperty:42};
let myVar2=myVar1;
myVar2.myProperty=0;
In this case myVar1 is set to reference the object created and the assignment copies the reference into myVar2. Notice that the content of myVar1 is copied in both cases, but when you zero myVar2.myProperty it is the same object as myVar1 references and so myVar1.myProperty is also zeroed.
The difference in behavior is not due to any change in the way assignment works, but due to what is being assigned. In the first case a value is stored in the variable and in the second a value which is a reference to the object is stored. This causes beginners lots of problems until they finally understand the idea of a reference to an object.
It is particularly confusing in parameter passing.
For example, continuing the previous example:
function myFunction(p1){
p1=0;
}
myFunction(myVar1):
In this case myVar1 isn't changed as JavaScript parameters are always passed by value and assigning to p1 has no effect on myVar1. This is true irrespective of whether myVar1 contains a value or a reference. However:
function myFunction(p1){
p1.myProperty=0;
}
myFunction(myVar1):
does change myProperty if myVar1 is an object that has that property. Notice that if myVar1 doesn't have the specified property the result is an error and this is the case even if myVar1 is a value such as 42.
In JavaScript all values are objects.
|