Page 2 of 3
Layout
Now that we have the web service installed we can build the user interface. Simply put three buttons, two text boxes, and a combo box onto the form.
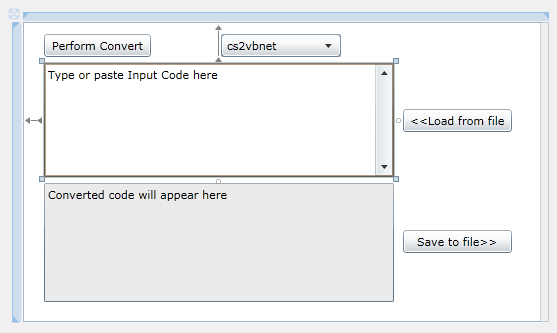
How these should be customised is fairly obvious but the XML that gives the layout actually used is:
<UserControl usual generated namespaces etc. d:DesignHeight="300" d:DesignWidth="518"> <Grid x:Name="LayoutRoot" Background="White" Width="526"> <TextBox Height="114" HorizontalAlignment="Left" Margin="21,41,0,0" Name="textBox1" VerticalAlignment="Top" Width="350" AcceptsReturn="True" VerticalScrollBarVisibility="Visible" HorizontalScrollBarVisibility="Auto" Text="Type or paste Input Code here "/> <TextBox Height="119" HorizontalAlignment="Left" Margin="21,161,0,0" Name="textBox2" VerticalAlignment="Top" Width="350" HorizontalScrollBarVisibility="Auto" VerticalScrollBarVisibility="Auto" IsReadOnly="True" Text="Converted code will appear here"/> <Button Content="Perform Convert" Height="23" HorizontalAlignment="Left" Margin="21,12,0,0" Name="button1" VerticalAlignment="Top" Width="107" Click="button1_Click" /> <ComboBox Height="23" Margin="198,12,0,0" Name="comboBox1" VerticalAlignment="Top" ItemsSource="{Binding}" HorizontalAlignment="Left" Width="120" SelectedIndex="0" DisplayMemberPath="Content"> <ComboBoxItem Content="cs2vbnet" /> <ComboBoxItem Content="vbnet2boo" /> <ComboBoxItem Content="vbnet2cs" /> <ComboBoxItem Content="cs2boo" /> </ComboBox> <Button Content="<<Load from file" Height="23" HorizontalAlignment="Left" Margin="380,87,0,0" Name="button2" VerticalAlignment="Top" Width="109" Click="button2_Click" /> <Button Content="Save to file>>" Height="23" HorizontalAlignment="Left" Margin="380,208,0,0" Name="button3" VerticalAlignment="Top" Width="109" Click="button3_Click" /> </Grid> </UserControl>
The most important property changes are that textBox1 has a vertical scroll bar and accepts carriage returns, textBox2 is readonly and the comboBox has a list of string items to display.
The code
The program doesn't do anything until the user clicks the Perform Convert button. The first thing is to get the selected conversion method from the comboBox
private void button1_Click( object sender, RoutedEventArgs e) { string type = (string) comboBox1.SelectionBoxItem;
Casting the comboBox item to a string is in general a risky operation but as we know for sure that they are in fact all strings - no problem. Next we get the contents of the textBox:
string Code = textBox1.Text;
To use the service we need an instance of ConvertServiceSoapClient:
ConvertServiceSoapClient converter = new ConvertServiceSoapClient();
We are going to call the ConvertAsync method which raises the ConvertComplete event when the conversion is complete. What this means is that we need to define a handler for the ConvertComplete event. We could go to the trouble of building a Delegate but its much simpler to use a lambda expression. All the lambda expression has to do is retrieve the result from the ConvertCompleteEventArg object that is passed to the handler:
converter.ConvertCompleted += new EventHandler<ConvertCompletedEventArgs>( (o,ConvertArgs) => { textBox2.Text=ConvertArgs.ConvertedCode; });
Now all that remains is to call the ConvertAsync method:
converter.ConvertAsync(type,Code); }
The complete Perform Conversion button's click handler is:
private void button1_Click( object sender, RoutedEventArgs e) { string type = (string) comboBox1.SelectionBoxItem; string Code = textBox1.Text; ConvertServiceSoapClient converter = new ConvertServiceSoapClient(); converter.ConvertCompleted +=new EventHandler<ConvertCompletedEventArgs> ( (o,ConvertArgs) => { textBox2.Text=ConvertArgs.ConvertedCode; }); converter.ConvertAsync(type,Code); }
<ASIN:0470524650 >
<ASIN:1430230185 >
<ASIN:0672333368 >
|