Page 1 of 2 Functions in Python are very important, even when you forget that they are objects. To make functions easier to use, there are a lot of additional features that have been added as Python developed. Parameters are explained in this extract from my book, Programmer's Python: Everything is an Object.
Programmer's Python Everything is an Object Second Edition
Is now available as a print book: Amazon
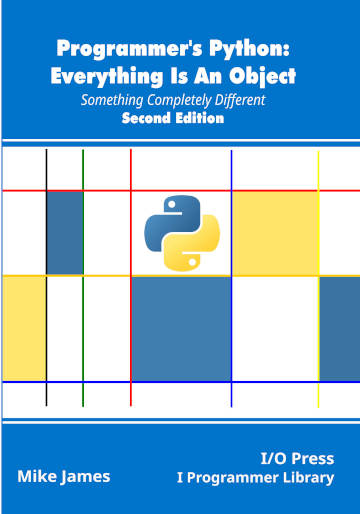
Contents
- Get Ready For The Python Difference
- Variables, Objects and Attributes
- The Function Object
- Scope, Lifetime and Closure
Extract 1: Local and Global ***NEW!
- Advanced Functions
- Decorators
- Class, Methods and Constructors
Extract 1: Objects Become Classes
- Inside Class
- Meeting Metaclasses
- Advanced Attributes
- Custom Attribute Access
- Single Inheritance
- Multiple Inheritance
- Class and Type
- Type Annotation
- Operator Overloading
- Python In Visual Studio Code
Extracts from the first edition
<ASIN:1871962749>
<ASIN:1871962595>
<ASIN:1871962765>

Default Parameters
The standard way of specifying parameters means you have to supply all of the parameters when you call the function. It is often a simplification to allow some parameters to have default values that are applied if the call doesn’t supply them.
Defaults are specified by assignment to the parameters in the function definition.
For example:
def sum(a=0,b=0):
return a+b
Now you can call the function as:
sum()
which sets both parameters to their default or:
sum(1)
which sets a to 1 and b to its default.
Notice that you can’t set b to a value and have a set to its default.
Defaults are generally set at the end of a parameter list.
You can use expressions to set defaults and these are evaluated just once, in left to right order, when the function is defined and not each time the function is called. This is a small but important point.
So for example:
i=0
def sum(a,b=i):
return a+b
i=1
print(sum(1))
i=2
print(sum(1))
prints 1 followed by 1. The local variable i is zero when the function is defined and any changes to it after the function has been defined are not relevant.
This is reasonable behavior as you would not expect the value of a default to depend on when the function was called.
Notice that even though the default is evaluated only once, if it is a mutable object like a List then it can be modified by the function and be different on each call.
What exactly happens is that the parameter list is evaluated and the defaults are stored in the __defaults__ attribute as a tuple.
For example:
def sum(a=0,b=1):
return a+b
print(sum.__defaults__)
prints (0,1).
You can assign a new tuple to __defaults__.
For example:
sum.__defaults__=(2,3)
print(sum())
prints 5.
It is difficult to think of a practical use of this facility but you never know.
Keyword Parameters
In addition to positional parameters, functions can be called using keyword parameters.
You don’t have to do anything in the function definition, simply assign the values to the parameter when you call the function.
For example:
sum(a=1,b=2)
you can assign in any order, all that matters is that there is a parameter in the function definition of the same name:
sum(b=2,a=1)
You can mix positional and keyword parameters but it is fairly obvious that positional parameters have to follow positional parameters, but see keyword-only parameters later.
Notice that keyword parameters can have default values.
|