Page 1 of 3 Type and Python is a difficult topic from a philosophical point of view. Python isn’t strongly typed and yet the predominate programming paradigm is class-based, strongly-typed. Before we can understand Python's approach we need to know what type is and why it relates to class. This is an extract from my book, Programmer's Python: Everything is an Object.
Programmer's Python Everything is an Object Second Edition
Is now available as a print book: Amazon
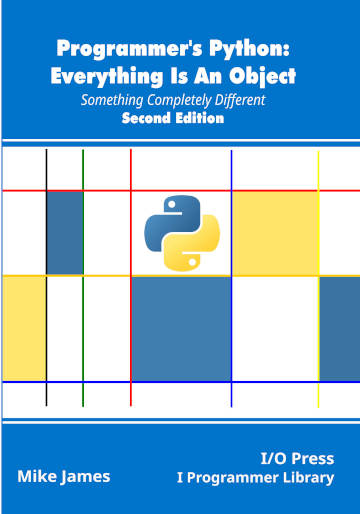
Contents
- Get Ready For The Python Difference
- Variables, Objects and Attributes
- The Function Object
- Scope, Lifetime and Closure
Extract 1: Local and Global
- Advanced Functions
- Decorators
- Class, Methods and Constructors
Extract 1: Objects Become Classes
- Inside Class ***NEW!
- Meeting Metaclasses
- Advanced Attributes
- Custom Attribute Access
- Single Inheritance
- Multiple Inheritance
- Class and Type
- Type Annotation
- Operator Overloading
- Python In Visual Studio Code
Extracts from the first edition

Some claim that Python is strongly typed because every object in Python has a type, which is the class or metaclass that created it as recorded in __class__. However, this is a very loose form of typing as it can be, like many things in Python, easily changed. All you have to do is assign another type to __class__.
In the same way variables are often said to be dynamically typed but as they can reference any Python object is seems more accurate to say that they are untyped.
In this chapter we look at the connection between class and type and how Python makes use of this.
Three Types Of Type
Even before we get started it is important to realize that there are a number of meanings to the word "type" in programming.
The most common usage of the word means primitive data type and after this it refers to the "class" that defines an object.
Exactly what these two meanings of type are all about will be explained in more detail later. For the moment it is assumed that you have a rough idea what a primitive type is, e.g. an int or a string, say, and you have an idea what a class-based type is, i.e. an instance of a class.
There is also a third meaning which corresponds to algebraic data type and this is a much deeper almost philosophical idea, the Howard Curry correspondence, that really doesn't have much to do with Python or the class-based languages that most of us know.
Such ideas of data typing are part of pure functional programming as found in languages such as Haskell and there is a lot to say about them, but it isn't really mainstream in the sense of languages like Python, JavaScript, Java, C++, etc.
For the rest of this chapter type will be taken to mean either primitive data type or class-based type. But we still have to say exactly what these are.
Primitive Data Types
Everything in Python is an object, but even in Python we cannot ignore the fact that some types of data are more primitive. In early computer languages all we had to work with was primitive data.
You could write:
a=3
b=3.14159
and:
c=”Hello World”
and these literals 3, 3.14159 and “Hello World” would be converted into some internal representation and stored in the variables concerned.
Storing the values in the variable gives rise to what is called value semantics which has largely given way to what Python uses – reference semantics. In value semantics the variable stores the value being worked with, in reference semantics the value is stored elsewhere and the variable stores a reference to it.
What really matters, however, is that the representations used for different types of data can be very different even if the data looks the same. That is in:
a=3
the 3 would be stored as a binary integer, a zero bit string ending 011. The number:
b=3.14159
would be stored in a completely different format – usually floating point. The difference between the two is quite large in that floating point can represent where the decimal point is, but the integer cannot.
|