Page 1 of 2 Without functions all we have are attributes of objects. Functions are where variables live. This extract from
Programmer's Python: Everything is an Object explains that functions bring something new to objects – local variables.
Programmer's Python Everything is an Object Second Edition
Is now available as a print book: Amazon
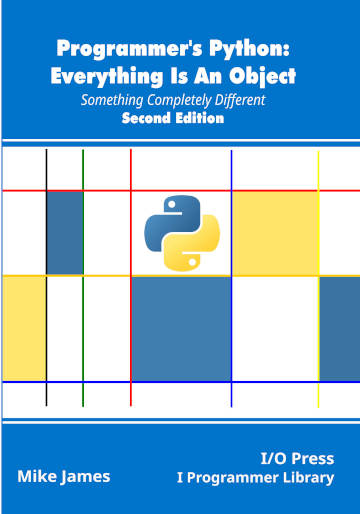
Contents
- Get Ready For The Python Difference
- Variables, Objects and Attributes
- The Function Object
- Scope, Lifetime and Closure
Extract 1: Local and Global
- Advanced Functions
- Decorators
- Class, Methods and Constructors
Extract 1: Objects Become Classes
- Inside Class ***NEW!
- Meeting Metaclasses
- Advanced Attributes
- Custom Attribute Access
- Single Inheritance
- Multiple Inheritance
- Class and Type
- Type Annotation
- Operator Overloading
- Python In Visual Studio Code
Extracts from the first edition

Functions bring something new – local variables.
Without functions all we have are attributes of objects. Functions bring something new to objects – local variables. These two, attributes and local variables, work together in a way that is cooperative, but you do have to keep in mind their differences. There is also an important difference between Python functions and most other language’s functions. Python functions are objects that exist even when the function is not being executed. This has some interesting consequences for the code that the function object is associated with.
Global v Local
You can define a function anywhere in a program. The simplest case is when a function is defined at the top level in a module. In this case all of the variables defined in the module are part of its execution context and are accessible from within the function. This is usually described as the function having access to the global variables of the module.
If you try to access a variable that isn’t defined as a local variable within the function, the variable table for the entity that the function is defined in is searched. If the variable is found then it is used, for example:
myGlobalVariable = 10
def myFunction():
print(myGlobalVariable)
myFunction()
In this case the print causes the system to look for myGlobalVariable in the local table. When it isn’t found the table of the containing entity, the module in this case, is searched and when the variable is found the value 10 is returned.
So far, so much like most languages. Python, however, works differently when storing a new reference in a variable. As Python has no keyword like var or let to signal that a new variable is being defined, an assignment to a variable always creates that variable unless it already exists as a local variable.
For example:
myGlobalVariable = 10
def myFunction():
myGlobalVariable = 0
print(myGlobalVariable)
myFunction()
print(myGlobalVariable)
makes an assignment to myGlobalVariable within the function. This has to create a new local variable of the same name and store 0 in it, which is what the function prints. The print after the function has completed displays 10 since the global variable is not affected by the local variable – which has now been destroyed because the function has ended. Recall that local variables only exist from the moment they are created until the function finishes executing.
In most languages assigning to a variable that didn’t exist locally but did exist globally would result in an assignment to the global variable. This cannot be how it works in Python because assignment creates local variables. So how do you assign to a global variable in Python? The answer is that you have to declare the variable global, for example:
myGlobalVariable=10
def myFunction():
global myGlobalVariable
myGlobalVariable=0
print(myGlobalVariable)
myFunction()
print(myGlobalVariable)
In this case the global modifier declares myGlobalVariable to be the same variable as in the enclosing entity, which means that the assignment doesn’t create a new local variable. The result is that the print in the function and the one after the function both display 0. The function has modified the global variable.
A subtle point is that if the variable doesn’t exist as a global then it will be created when it is assigned to. That is, global allows you to create global variables from within functions.
You can declare a list of variables as global:
global var1, var2, var3 ...
and all of these variables will be treated as global. The global declaration has to occur in the code before any assignments to the variable which would create a local variable of the same name.
Python does global and local the other way round compared to most languages but in most cases you want to make use of local variables and accessing globals is the exception.
|