Page 1 of 4 jQuery provides both a general framework for handling events and event-specific functions. While you can use the general functions - on, off and one - to deal with all events, the event-specific functions do focus the mind on how events are used and are worth knowing about.
Just jQuery Events, Async & AJAX
Is now available as a print book: Amazon
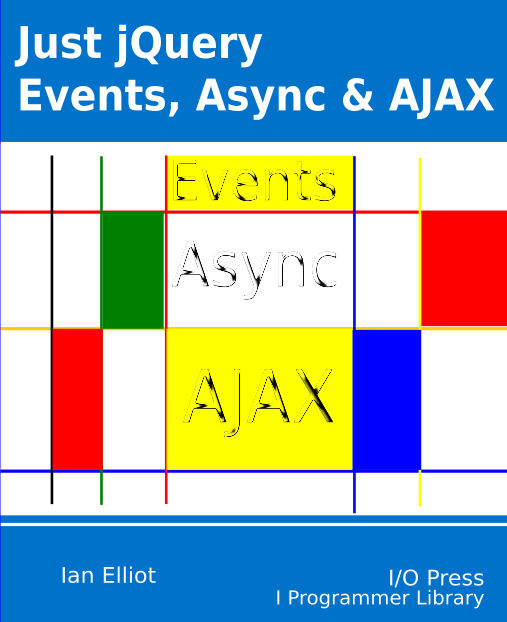
Contents
- Events, Async & Ajax (Book Only)
- Reinventing Events
- Working With Events
- Asynchronous Code
- Consuming Promises
- Using Promises
- WebWorkers
- Ajax the Basics - get
- Ajax the Basics - post
- Ajax - Advanced Ajax To The Server
- Ajax - Advanced Ajax To The Client
- Ajax - Advanced Ajax Transports And JSONP
- Ajax - Advanced Ajax The jsXHR Object
- Ajax - Advanced Ajax Character Coding And Encoding
Also Available:
buy from Amazon
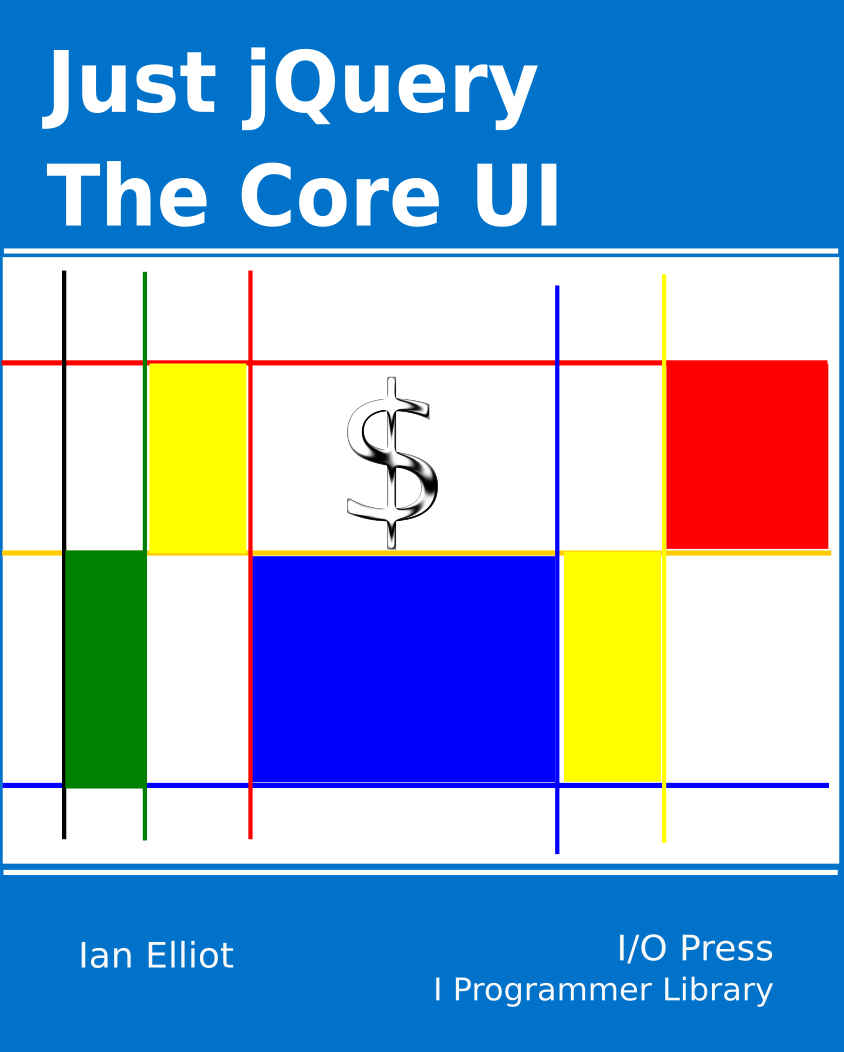
Advanced Attributes
Events are central to the way we program in JavaScript. A JavaScript program is essentially a collection of event handlers. In this chapter we take a closer look a the different types of event you are likely to encounter and in particular the events that jQuery thinks are important enough to be worth having special functions for, in addition to the general purpose on, off and one, which switch an event on just once.
There are five categories of event:
- Mouse
- Keyboard
- Form
- Browser
- Document
Keep in mind that all of these functions are equivalent to using on with the name of the event. This means that events that are attached using these functions can be manipulated as if they had been added using on. For example they can be removed using off.
Mouse Events
Click
Mouse events are the ones we use most. The first example of an event most people are introduced to is the mouse click event. However the mouse can be a difficult input device to get right.
The most basic mouse event is the click and you can attach a handler to any element using:
click(eventdata, handler)
where eventdata is optional and is an object that is passed to the handler when it is fired. You can also fire the click event on an element by using
click()
i.e. with no parameters.
All of the specific event functions work in the same way - only the name of the event and function changes.
For example:
$("#button1").click(function(event){ console.log("Button1 clicked"); });
We are so familiar with the click event that we often don't bother to find out exactly what a click is. To register a click the left mouse button has to to be pressed down and released while the mouse pointer is inside the element that registers the click. What this means is that you can abort a click by moving outside of the element before you release the button.
There are lower level mouse event handlers mousedown and mouseup which are fired when a mouse button is pressed and when released respectively. A mousedown and a mouseup event occur before a click event.
A click event handler will be called when a user taps on a touch device and so it is fairly safe to use.
Double Click
There is also the double click event and dblclick function that can be used to attach a handler in much the same way:
$("#button1").dblclick(function(event){ console.log("Button1 double clicked "); });
In this case the surprise might be that you can double-click a button. It may be allowed but it isn't used very often. In fact as the double click generally means "open me" or "run me" it tends to only be used on elements that can be opened or run.
A double click is slightly more complicated than a click because there is a system specific delay between clicks. The double click event is fired when the user clicks twice on an element within a given time. As for a click the pointer has to remain inside the element the entire time.
Double clicks are best avoided if you want your program to work on a mobile device. The reason is that there is no direct equivalent to the double click. A single tap may be treated as a click but a double tap is not and in fact double tapping is a very rare gesture on a touch-enabled app. If you really must have a double click working on a mobile device then you will need to write something at a lower level and this is difficult to get working across devices.
Another problem is that browsers differ in the way they handle click and double click handlers bound to the same device - some fire two click events before the double click and some just one.
Finally notice that click and double click only work with the mouse's primary button - usually the left button. Some browsers did fire a click event for other buttons but this is not now standard behaviour.
If click and double click is only for the primary button what about the middle/scroll wheel and the right button?
Right Click
The right button is associated with the context menu and so its event is called contextmenu.
$("#button1").contextmenu(function(event){ console.log("Button1 right clicked "; });
The event will only fire if the button is right clicked. The event handler will be called but when it has finished the context menu will be displayed. To suppress the context menu you need to add a call to preventDefault:
$("#button1").contextmenu(function(event){ console.log("Button1 right clicked "); event.preventDefault(); });
Long touch events are generally translated to right clicks, i.e. the display the context menu in a mobile device. For this reason using right click is relatively safe.
mouseup and mousedown
The middle button or scroll wheel doesn't have a special event handler in jQuery and only recently has there been a standard for its use. If you want to work with the scroll button then look up the "wheel" event.
If you want to respond to left, middle and right clicks then you have no choice but to program your own. The lower level events mousedown and mouseup are fired when any mouse button is used and you can discover which button was pressed using the which property of the event object, which is 1 for the left button, 2 for the middle button and 3 for the right button.
For example:
$("#button1").mousedown(function(event){ console.log("Button1 down "+event.which); event.preventDefault(); });
in this case you will see 1,2 or 3 displayed depending on which button you press.
You can use mousedown and mouseup to synthesise a click event on any button, however it isn't quite as easy as you might think. If you want to make a proper click event then you have to make sure that the mouseup happens on the same element that the mousedown occurred on. One way of doing this is:
var clickdown = function (event) { event.preventDefault(); var target = event.target; $(document).on("mouseup", function (event) { if (target === clickdown.target) { console.log("click " + event.which); } $(document).off("mouseup"); }); };
What is happening here is that when the mousedown event occurs the element that it occurred on is stored in the the local variable target. Then the function sets a mouseup event handler on the document. Any mouseup event that occurs will bubble up to the top level document and we can check using target, which is available to the handler due to closure, that the event did occur on the same element.
To use this click function all you have to do is:
$("#button1").mousedown(clickdown);
Notice that the actual event handler, i.e. the code that does something useful, is defined on the mouseup handler.
Mouse Location Events
A set of events is provided to let you respond to a mouse's location. Notice that none of these events have good equivalent gestures on a mobile or touch device and hence they are best avoided.
mouseenter and mouseleave
Are triggered when the mouse pointer enters and leaves an element respectively. This event doesn't bubble so the mouse entering and leaving a child element does not fire the event on the element containing the child.
mouseover and mouseout
Are triggered when the mouse pointer enters and leaves an element respectively. They are bubbling versions of mouseenter and mouseleave. This means that the events will be fired if the mouse pointer enters or leaves a child element of the element the handler is bound to.
To see the difference use something like:
<div id="outer"> Outer <div id="inner"> Inner </div> </div>
and
$("#outer").mouseover(function () { console.log("enter"); });
You will see the event triggered if the mouse moves over the inner or outer div. If you change mouseover to mouseenter you will only see the event when the mouse moves over the inner element.
hover
Hover will bind one or two handlers to the mouseenter and mouseleave events. If you specify two functions then the first is bound to mouseenter and the second to mouseleave. If you specify a single function then it is bound to both events.
mousemove
This event is fired every time the mouse moves within the element the handler is bound to. You can obtain the mouse's position from the pageX and pageY properties of the event object. These give the position relative to the top left-hand corner of the page. The only problem with using this event is that it is fired every time the mouse moves and this can be a performance issue. Any event handler you bind to it should finish as quickly as possible.
As an example of mousemove, let's implement a "scribble" application. In this case all we have to do is draw a dot at the current mouse position as it moves. To do this we can use a canvas element:
<canvas id="Canvas" width="600" height="600" style="height:600px;width:600px;"> </canvas>
The program is:
$(document).mousemove(function (event) { var c = $("#Canvas"); var ctx = c[0].getContext("2d"); ctx.fillStyle = "rgb(200,0,0)"; ctx.fillRect(event.pageX, event.pageY, 1, 1); });
If you try this out you should be able to drawn on the page
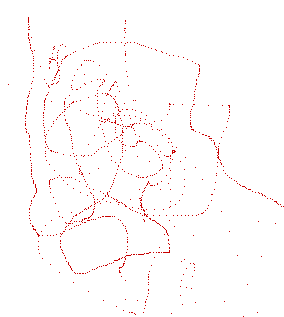
If you change mousemove to mousedown then you will only be able to draw at each mousedown event.
|