Page 1 of 4 Sometimes it is useful for an object to have a default value so that it can be used in an expression such as object+1 and object+"hello world". In JavaScript this is really easy and it is an important general principle.
Now available as a book from your local Amazon.
JavaScript Jems: The Amazing Parts
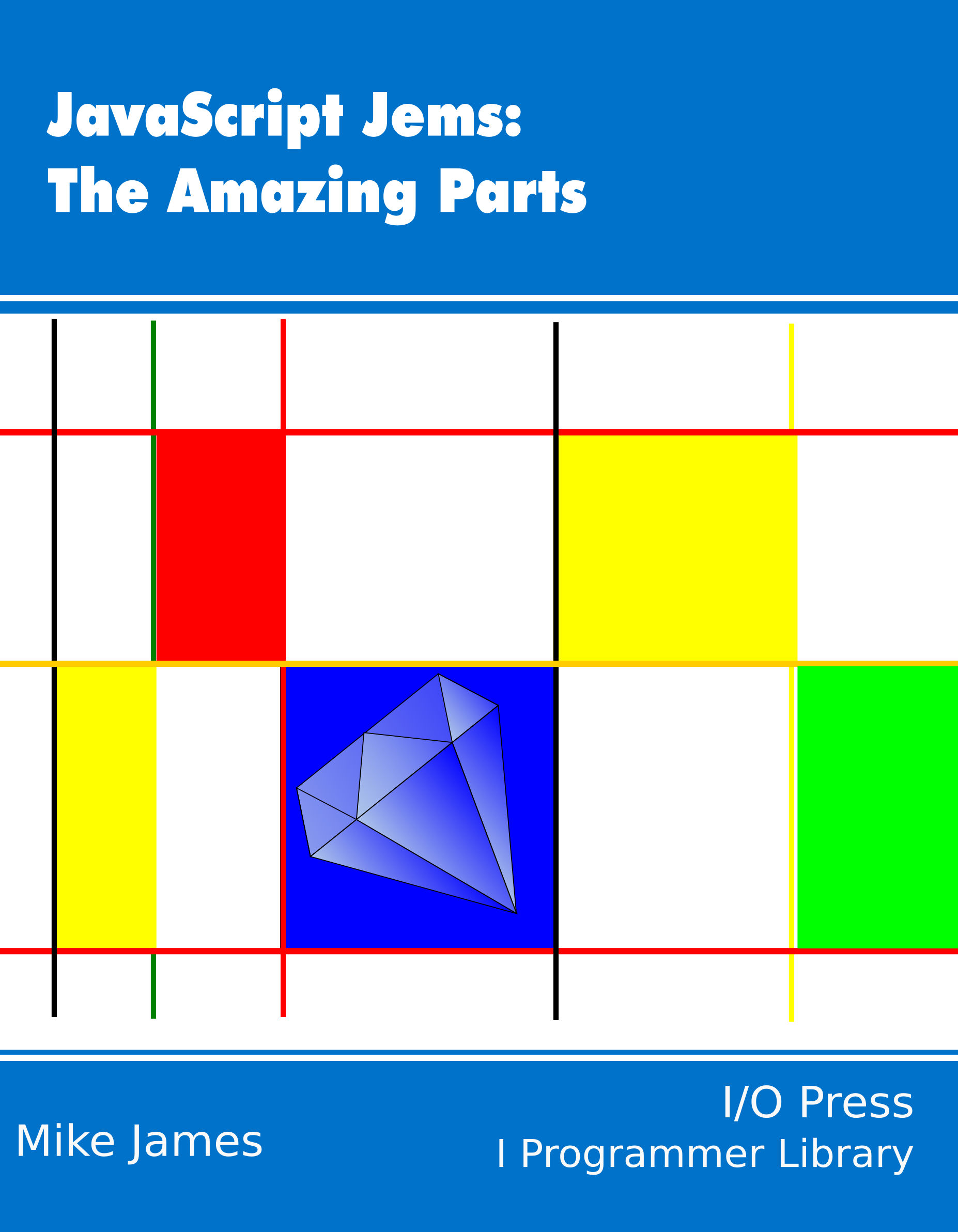
JavaScript Jems - Functional And Not Quite Functional
Original drafts of chapters.
Contents
- JavaScript Patterns
Why JavaScript is a Jem
- Objects with Values in JavaScript*
- Private Functions In JavaScript
- Chaining - Fluent Interfaces In JavaScript*
- Active Logic, Truthy and Falsey*
- The Confusing Comma In JavaScript*
- Self Modifying Code*
- Lambda expressions
- Meta Programming Using Proxy
- Master JavaScript Regular Expressions
- The Function Approach To Programming
<ASIN:1871962579>
<ASIN:1871962560>
<ASIN:1871962501>
<ASIN:1871962528>
Sometimes it is good for an object to have a value, or even more than one value. For example, suppose you have an object that represents an item in an order. The object may have a number of additional properties and methods.
For example:
item.name="widget"; item.tax=0.1; item.price=10;
However if you consider the primary property of an item to be its price you might want to write expressions such as:
totalcost=item1+item2;
to mean
totalcost=item1.price+item2.price;
Similarly you might consider the item's name to be its primary string property and you might want to write statements like:
alert(item);
to mean
alert(item.name);
You might argue that this isn't a good example of using default values and that it would be better to use the full property names. However, there are examples where it is natural for the values stored in an object to take part in arithmetic, logical or string expressions and in these cases not to have the ability to define default values.
For example the JavaScript Date object returns the date as the number of milliseconds from the start date when used as if it was a number and a formatted date string when used as a string. Some objects really are more like data than method.
So how do you define a default value?
The answer is surprisingly simple.
When an object is involved in an expression the valueOf method is called which returns a numeric or Boolean value. The numeric or Boolean value is further type converted to make the expression work. If the object is part of an expression where a string would be required then toString is called.
So far this is nothing new and it just gives rise to the type conversion rules that you should know about. However you can define your own valueOf and toString methods and these can be used to deliver custom default values.
valueOf
All JavaScript objects will supply a value when asked for one. In many cases the value isn't of much use and hence it tends to be overlooked as a useful feature.
For example:
var myObject={ valueOf:function(){ return 20; } };
Following this you can write:
var result=myObject*10;
and the result will be 200.
If an object has a valueOf function defined then it can be used to supply a value whenever the object is used in an expression and a numeric value is required.
What do you think you get if you write the simpler:
var result=myObject;
The answer is not that result is set to the simple value 20. This is an object assignment and result is set to another reference to myObject. This can be confusing because if you now try something like:
var a=result*10;
you will still see 200 stored in a because result refers to the same object as myObject and so valueOf is called to return 20 in the expression. In other words result behaves as if it was 20 in an expression just as myObject does. However, if anything changes myObject so that it returns some other value, then a will not equal 200 after the expression.
It also works if a Boolean value is required. For example, if you change the definition of myObject to:
var myObject={ valueOf:function(){ return true; } };
then you can write things like
!myObject
which evaluates to false.
At this point you might be wondering how you can work out what to return from valueOf when you can't know how it will be used in an expression. For example, what if you you use the Boolean valued myObject in an arithmetic expression?
Of course, there is nothing to worry about because JavaScript's type juggling will sort it out for you and true will be converted to the value 1. In the same way returning a default value of 1 will be treated as true within a Boolean expression.
This is very flexible, but you should always return a value that is appropriate for the meaning of the object you are working with.
|