Page 1 of 2 The functional approach to iteration is probably the main reason that programmers are initially attracted to the idea of functional programming. This is an extract from my newly published book, JavaScript Jems: The Amazing Parts.
Now available as a book from your local Amazon.
JavaScript Jems: The Amazing Parts
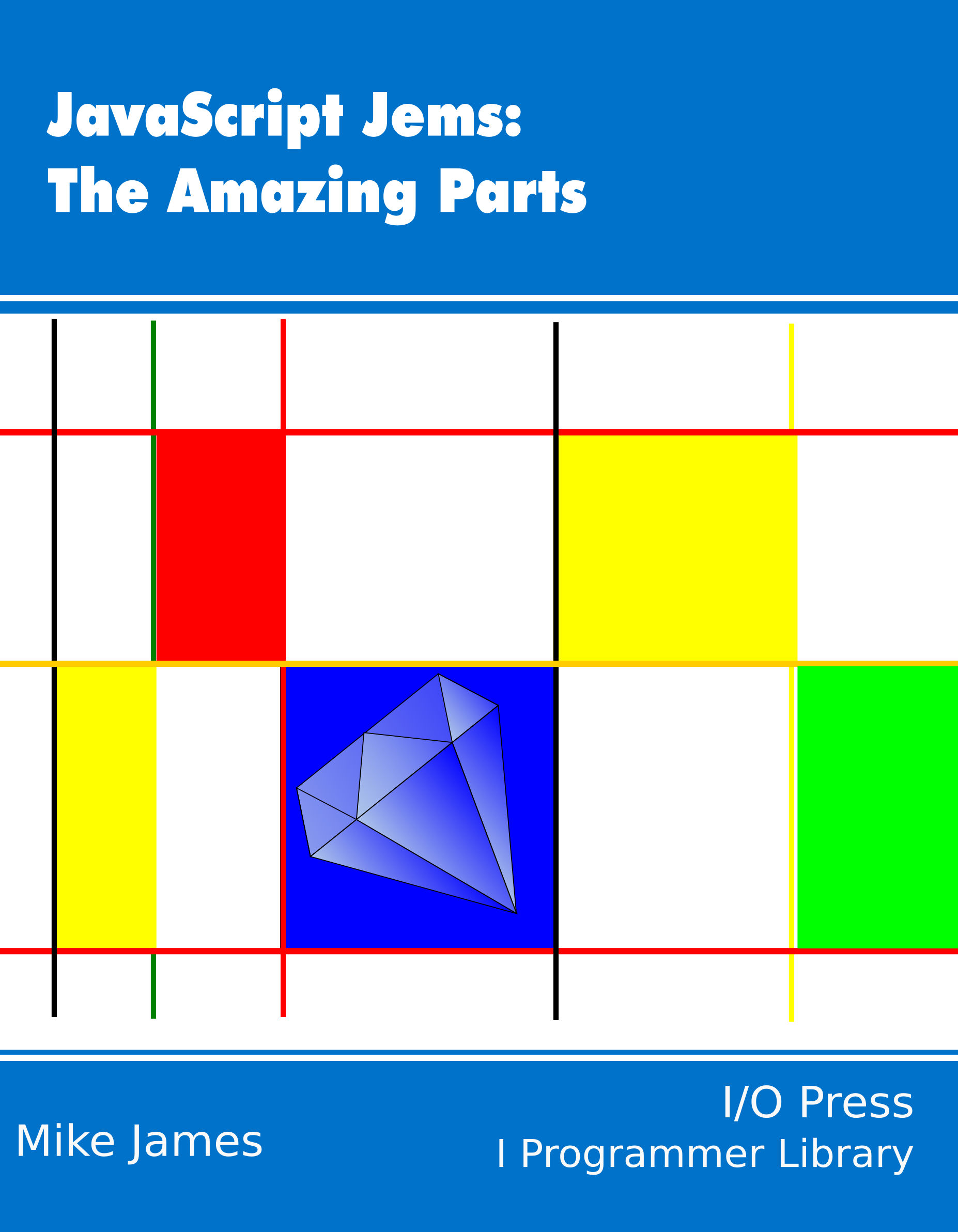
Contents
Jem 18 Functional Approach To Iteration
“Few ideas work on the first try. Iteration is key to innovation.”
Sebastian Thrun
Using functional iteration is a compact and direct way to express the idea of doing something with a collection of data entities and it leads on naturally to fluent interfaces, which share many of the same characteristics. It also generalizes to the idea of using functions, even if they are impure and don't conform well to the broader tenets of functional programming simply because they express the intent of the program better than the equivalent control structures. This utilitarian approach to using functions isn't quite functional programming and you might as well call it function-oriented programming or FOP.
Let's see how all this arises from the familiar Array object.
Arrays and For Loops
Arrays are the simplest of data structures and they pair perfectly with the for loop, providing us with the first example of a data structure determining the program structure used to process it. If you have an Array A then each element is indexed by an integer and to process the elements of the Array all you have to do is iterate the index using a for loop:
const myArray=["a","b","c"];
for(let i=0;i<3;i++){
console.log(myArray[i].toUpperCase());
}
You can see that the idea is that we repeat the operation on the Array for i set to 0, 1 and 2 - we "step through" the array processing it as we go.
In many cases the index i and the element myArray[i] are both needed, but sometimes you just need the element myArray[i]. In such cases you can use the for of loop. which steps through an array in the same order as the index:
const myArray=["a","b","c"];
for(const element of myArray){
console.log(element.toUpperCase());
}
In the case where you only want the index, you can use a for in loop:
const myArray=["a","b","c"];
for(const index in myArray){
console.log(index));
}
We’ll look at why you might want to do this later.
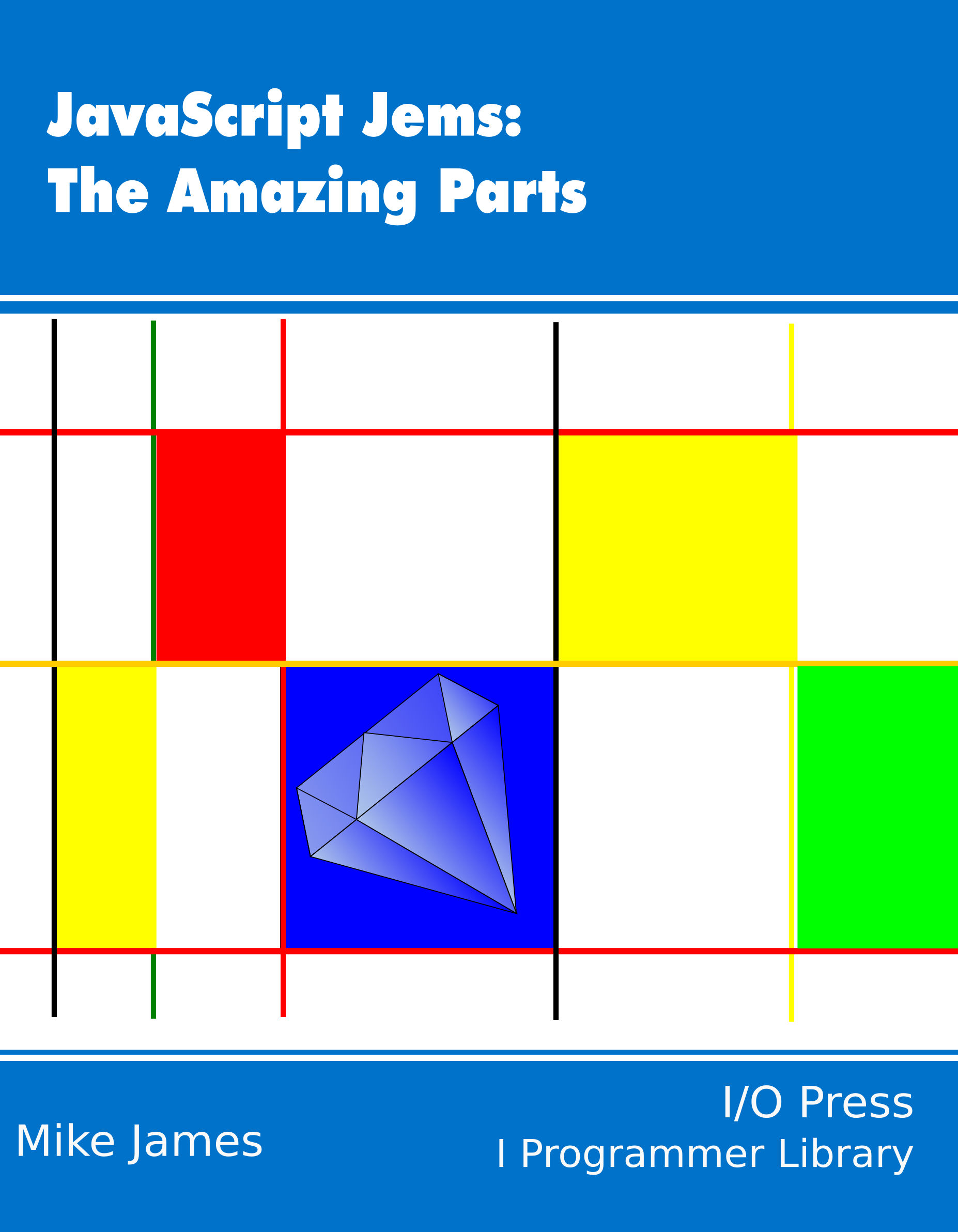
Functional For
For loops and arrays go together, but there is a way of packaging for that makes it disappear. Array has a forEach method that will apply a function that you supply to each element in an array. The function is supplied with three arguments - the current element, the index and the entire array. All of the parameters are optional and usually only element and perhaps index are used. For example:
const myArray = ["a", "b", "c"];
myArray.forEach(function (element)
{
console.log(element.toUpperCase());
}
);
does the same job as the for loop and the for of loop in the previous section. It looks easier to understand and if you write it using an arrow function it is even more compact:
myArray.forEach( element => console.log(element.toUpperCase()) );
Many beginners are impressed by forEach, or one of the many similar functions, and they tend not to ask "where did the for loop go?" The answer, however, is fairly obvious and you can see that the forEach method is just equivalent to:
function forEach(f){
for(const index in myArray){
f(this[index],index,this);
}
}
So it isn't mysterious or even clever, but it is attractive to use.
There are Array methods that hide for loops and the best known is map which works like forEach, but creates a new array using the return value of the function. For example:
const myArray = ["a", "b", "c"];
const myArray2 = myArray.map(function (element) {
return element.toUpperCase();
}
);
console.log(myArray2);
Notice that in this case the map method returns a new Array with the result, in the spirit of immutability. The function that is applied to each element has to return the new value of each element. Don't use map if you don't modify the array as it is just a waste of memory to create a new array you don't want.
|