Page 1 of 3 It is clear that JavaScript is a subtle and sophisticated language that deserves to be treated in its own right and not as a poor copy of other object-oriented languages. JavaScript doesn’t have type so the question is how do we cope?
This is an extract from the book Just JavaScript by Ian Elliot.
Buy Now: from your local Amazon
Just JavaScript An Idiomatic Approach
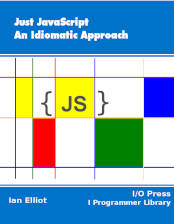
A Radical Look At JavaScript
Most books on JavaScript either compare it to the better known class based languages such as Java or C++ and even go on to show you how to make it look like the one of these.
Just JavaScript is an experiment in telling JavaScript's story "just as it is" without trying to apologise for its lack of class or some other feature. The broad features of the story are very clear but some of the small details may need working out along the way - hence the use of the term "experiment". Read on, but don't assume that you are just reading an account of Java, C++ or C# translated to JavaScript - you need to think about things in a new way.
Just JavaScript is a radical look at the language without apologies.
Contents
- JavaScript – Essentially Different
- In The Beginning Was The Object
- Real World Objects
- The Function Object
Extract - The Function Object Extract - Function Object Self Reference
- The Object Expression
- Function Scope, Lifetime & Closure
Extract Scope, Lifetime & Closure Extract Execution Context ***NEW!
- Parameters, Returns and Destructuring
Extract - Parameters, and Destructuring
- How Functions Become Methods
- Object Construction
Extract: - Object Factories
- The Prototype
Extract - ES2015 Class and Extends
- Inheritance and Type
- The Search For Type
- Property Checking
Buy Now: from your local Amazon
Also by Ian Elliot JavaScript Async: Events, Callbacks, Promises and Async Await Just jQuery: The Core UI Just jQuery: Events, Async & AJAX
Many programmers are so certain that they need a strong type system that they attempt to find it in JavaScript.
There are some facilities that seem to provide some sort of replacement for the idea of type and inheritance, and these are the subject of this chapter.
In particular we look at the constructor and its associated prototype object as substitutes for an object's type and how instanceof attempts to provide something like subtypes.
There are so many problems with all of these approaches that, to an extent, the purpose of this chapter is to point out how they fail so that if you use them you know what to expect.
Mostly all of the facilities in this chapter are best avoided unless you really understand them and have a use for them.
In the next chapter we look at realtime type checking and some alternatives to typing.
Prototypical Inheritance
Final version in book
The Constructor As An Object’s "type"
Every JavaScript object is associated with two important objects - its constructor and its prototype, and both play a role in attempts to apply the ideas of type to JavaScript.
The constructor is the first related object we need to consider because a constructor usually creates multiple identical objects. In this sense a constructor creates multiple instances of the abstract object it was designed to create, and even though it is an object it plays the role of a class.
In fact this cozy picture of a constructor stamping out identical objects isn't necessarily true - but let's assume it is for the moment.
Even object literals can be considered to have Object as their constructor and Object.prototype as their prototype.
If you know an object's constructor then you know the minimum set of properties and methods an object supports, i.e. all of those added by the constructor and all of those added by the prototype chain set by the constructor.
So there is a very strong sense that the "type" of an object is related to its constructor. If you know an object’s constructor then you can be fairly certain it has a given set of properties, especially if it is frozen or sealed.
Even if you allow dynamic ad-hoc properties, you can still assume that objects created by the constructor have the same minimum set of properties. It is only when you start using the delete operator that things go wrong.
However, even if you do ban the delete operator this isn't absolutely true because a constructor could conditionally add properties.
For example:
var C=function(){
this.x=10;
if(Math.random()<0.5){
this.y=20;
}
}
This creates an object which always has a property x but only has a property y 50% of the time. So if you discover that obj has been created by C you can, delete not withstanding, safely assume that it has a property x but not that it has a property y.
Such constructors are perverse but they are still valid JavaScript.
At the end of the day knowing an object's constructor is still your best guide to what properties it should have, but it is far from perfect.
As long as you make the rule that a constructor always creates objects with the same set of properties then it seems reasonable to use the name of the constructor as if it was the object's type.
So, borrowing from class-based language jargon, we can say that:
This isn't good jargon because o and C are two very different objects and to say o is an instance of C suggests that it is somehow similar but it is jargon you will find commonly used.
A much bigger problem is still that the constructor doesn’t have an immutable name, only variable references.
|