Page 4 of 4
The Simple HTTPS Client Listing
Putting all this together we need to create a project with the following files:
main.c full listing below
lwipopts.h add the lines given earlier to the examples
lwipopts file
mbedtls_config.h full listing given earlier
pico_sdk_import_cmake unmodified standard file in all Pico projects
CmakeLists.txt full listing given earlier
setupWiFi.h standard connection file given earlier
The full main.c is:
#include <stdio.h>
#include "pico/stdlib.h"
#include "pico/cyw43_arch.h"
#include "lwip/altcp.h"
#include "lwip/altcp_tls.h"
#include "setupWifi.h"
#define BUF_SIZE 2048
char myBuff[BUF_SIZE];
char header[] = "GET /index.html HTTP/1.1\r\n
HOST:example.com\r\n\r\n";
err_t recv(void *arg, struct altcp_pcb *pcb, struct pbuf *p, err_t err) {
if (p != NULL) { printf("recv total %d this buffer %d next %d err %d\n", p->tot_len, p->len, p->next, err); pbuf_copy_partial(p, myBuff, p->tot_len, 0); myBuff[p->tot_len] = 0; printf("Buffer= %s\n", myBuff); altcp_recved(pcb, p->tot_len); pbuf_free(p); } return ERR_OK; }
static err_t altcp_client_connected(void *arg, struct altcp_pcb *pcb, err_t err) { err = altcp_write(pcb, header, strlen(header), 0); err = altcp_output(pcb); return ERR_OK; }
int main() { stdio_init_all(); connect(); struct altcp_tls_config *tls_config = altcp_tls_create_config_client(NULL, 0); struct altcp_pcb *pcb = altcp_tls_new(tls_config, IPADDR_TYPE_ANY); mbedtls_ssl_set_hostname(altcp_tls_context(pcb), "example.com"); altcp_recv(pcb, recv); ip_addr_t ip; IP4_ADDR(&ip, 93, 184, 216, 34); cyw43_arch_lwip_begin(); err_t err = altcp_connect(pcb, &ip, 443, altcp_client_connected); cyw43_arch_lwip_end(); while (true) { sleep_ms(500); } }
In chapter but not in this extract:
Non-blocking HTTPS Request
Summary
-
Public key cryptography works with two keys, a private key and a public key, and hence is called asymmetric key cryptography. The public key is not secret and can be used by anyone to encrypt a text. The encrypted text can only be decrypted using the private key which is kept secret.
-
Symmetric key cryptography uses a single key which has to be kept private to the sender and receiver to encrypt and decrypt text.
-
Symmetric key cryptography is much faster than asymmetric and so what happens is that asymmetric keys are used to establish a single secret symmetric key that both the client and server use.
-
A certificate contains identity information and keys.
-
A client and a server can establish encrypted communication in one of two ways. If both have a certificate then the keys are used to exchange a single symmetric key. If only the server has a certificate then this is used by both parties to construct a shared secret key.
-
SSL, which later evolved into TSL, is used to add encryption to sockets. The lwIP RAW doesn’t use sockets but it can still make use of TLS to implement HTTPS via ALTCP.
-
To implement TLS you need to use ALTCP and the mbedtls library. The connection between the two is the altcp_tls library.
-
To configure mbedtls you need to use the mbedtls_config.h file to define the encryption methods you want to use.
-
The most commonly encountered methods are RSA key exchange followed by AES symmetric encryption.
-
Putting all this together it is easy to create an HTTPS client without the need to work with certificates.
Master the Raspberry Pi Pico in C: WiFiwith lwIP & mbedtls
By Harry Fairhead & Mike James
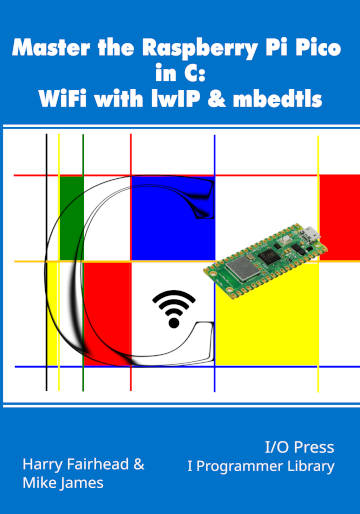
Buy from Amazon.
Contents
Preface
- The Pico WiFi Stack
- Introduction To TCP
Extract: Simplest HTTP Client
- More Advanced TCP
- SSL/TLS and HTTPS
Extract: Simplest HTTPS Client
- Details of Cryptography
Extract: Random Numbers
- Servers
Extract: HTTP Server NEW!!
- UDP For Speed
Extract: Basic UDP
- SNTP For Time-Keeping
- SMTP For Email
- MQTT For The IoT
Appendix 1 Getting Started In C
<ASIN:B0C247QJLK>
|