Page 4 of 4
The CMakeLists.txt file is as before:
cmake_minimum_required(VERSION 3.13)
set(PICO_BOARD pico_w)
set(CMAKE_C_STANDARD 11)
set(CMAKE_CXX_STANDARD 17)
include(pico_sdk_import.cmake)
project(PicoW C CXX ASM)
pico_sdk_init()
add_executable(main
main.c
)
target_include_directories(main PRIVATE ${CMAKE_CURRENT_LIST_DIR})
target_link_libraries(main pico_stdlib pico_cyw43_arch_lwip_threadsafe_background)
pico_add_extra_outputs(main)
and you also need the default lwipopts.h, pico_sdk_import.cmake. launch.json and of course setupWifi.h given in a previous chapter - but listed below for completeness:
int setup(uint32_t country, const char *ssid, const char *pass, uint32_t auth, const char *hostname, ip_addr_t *ip, ip_addr_t *mask, ip_addr_t *gw) { if (cyw43_arch_init_with_country(country)) { return 1; } cyw43_arch_enable_sta_mode(); if (hostname != NULL) { netif_set_hostname(netif_default, hostname); } if (cyw43_arch_wifi_connect_async(ssid, pass, auth)) { return 2; }
int flashrate = 1000; int status = CYW43_LINK_UP + 1; while (status >= 0 && status != CYW43_LINK_UP) { int new_status = cyw43_tcpip_link_status( &cyw43_state, CYW43_ITF_STA); if (new_status != status) { status = new_status; flashrate = flashrate / (status + 1); printf("connect status: %d %d\n", status, flashrate); } cyw43_arch_gpio_put(CYW43_WL_GPIO_LED_PIN, 1); sleep_ms(flashrate); cyw43_arch_gpio_put(CYW43_WL_GPIO_LED_PIN, 0); sleep_ms(flashrate); } if (status < 0) { cyw43_arch_gpio_put(CYW43_WL_GPIO_LED_PIN, 0); } else { cyw43_arch_gpio_put(CYW43_WL_GPIO_LED_PIN, 1); if (ip != NULL) { netif_set_ipaddr(netif_default, ip); } if (mask != NULL) { netif_set_netmask(netif_default, mask); } if (gw != NULL) { netif_set_gw(netif_default, gw); } printf("IP: %s\n", ip4addr_ntoa( netif_ip_addr4(netif_default))); printf("Mask: %s\n", ip4addr_ntoa( netif_ip_netmask4(netif_default))); printf("Gateway: %s\n", ip4addr_ntoa( netif_ip_gw4(netif_default))); printf("Host Name: %s\n", netif_get_hostname(netif_default)); }
return status; }
int connect()
{
char ssid[] = "ssid”; char pass[] = "password"; uint32_t country = CYW43_COUNTRY_code; uint32_t auth = CYW43_AUTH_WPA2_MIXED_PSK; return setup(country, ssid, pass, auth, "MyPicoW", NULL, NULL, NULL); }
If you run the program you will see the headers and HTML that makes up the page at example.com. You will also notice that the page is sent using more than one packet.
Many improvements could be included to finish this simple program, but first we need to convert the program from the TCP module to the Application Layered module.
In book but not in this extract
- The Application Layered Module
- A More Practical Client
Summary
-
Networks transfer data between devices as packets, complete with source and destination address.
-
Ethernet is a low-level protocol which is used to transfer packets between machines connected to a local network. Each machine has a unique MAC address which is used to determine where a packet should be sent.
-
Ethernet isn’t a routable protocol in the sense that MAC addresses give no clue as to where a machine might be located.
-
IP is a routable protocol which means it can be used to send packets to distant networks.
-
IP is usually transferred within Ethernet packets and IP addresses are converted to MAC addresses using ARP. If an IP address is not found on the local network then the packet is sent to a router, which passes it on to other networks.
-
The IP protocol is used to implement higher-level protocols such as UDP and TCP.
-
In their turn these higher-level protocols are used to implement protocols which transfer larger units of data such as HTTP which uses TCP to transfer web pages.
-
The lwIP library is structured according to the same protocol levels into modules. As we are not using Free RTOS, the RAW API is the one to use and it works in terms of callbacks.
-
The TCP module works in terms of PCBs Protocol Control Blocks to specify the callbacks to be used and the state of the connection.
-
The basic procedure is to create and initialize a PCB with at least a receive callback defined then connect to a specified IP and port address and supply a connected callback to send data back to the server. The receive callback then processes the data.
-
HTTP defines actions such as GET and PUT which retrieve a file from the server or send a file to the server respectively.
-
If you want to modify the connection protocol, e.g. replace HTTP by HTTPS then you should use the Application Layer TCP which works in the same way as the TCP module.
Master the Raspberry Pi Pico in C: WiFiwith lwIP & mbedtls
By Harry Fairhead & Mike James
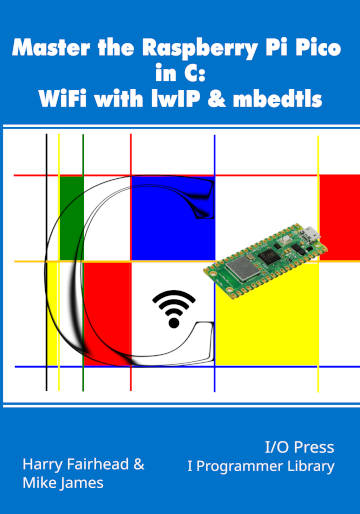
Buy from Amazon.
Contents
Preface
- The Pico WiFi Stack
- Introduction To TCP
Extract: Simplest HTTP Client
- More Advanced TCP
- SSL/TLS and HTTPS
Extract: Simplest HTTPS Client
- Details of Cryptography
Extract: Random Numbers
- Servers
Extract: HTTP Server NEW!!
- UDP For Speed
Extract: Basic UDP
- SNTP For Time-Keeping
- SMTP For Email
- MQTT For The IoT
Appendix 1 Getting Started In C
|