Page 2 of 4
Drawables
Drawables are slightly different from other resources in that there is no XML file that defines them. To create and use a drawable all you have to do is copy the graphic file that you want to use into the drawable/ directory. You don't need XML to define the resource because the resource's id is just the file name.
The best way to explain how drawable resources work is via a simple example. If you want to create a jpg bitmap resource all you have to do is copy the jpg file into the correct resource directory. Android Studio automatically creates the drawable/ directory for you and all you have to do is copy the bitmap file into it - but how?
The first thing to say is that the file name for a drawable resource can only contain lower case letters and digits. If the original file name doesn't conform to this pattern you can rename it after you have copied it into the directory.
There are two fairly easy ways:
You can find the file in the usual file directory structure and use copy and paste to paste it into the directory displayed in Android Studio.
Alternatively you can right click on the drawables folder in Android Studio and select Show in Explorer which opens the folder as a standard file system folder to which you can copy the bitmap file in any way that you like, drag-and-drop for instance.
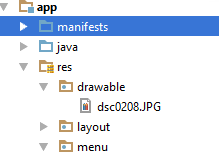
Once you have the file in the drawable/ directory you can make use of it.
Place an ImageView control on the UI using the designer. The Resources window opens automatically for you select the drawable you want to use. If you want to change the drawable at a later time find its src property in the Properties window and click on the ... button at the far right and the Resources window will open.
Select the Project tab and scroll down until you can see the name of the file you have just copied into the drawable folder and select it.
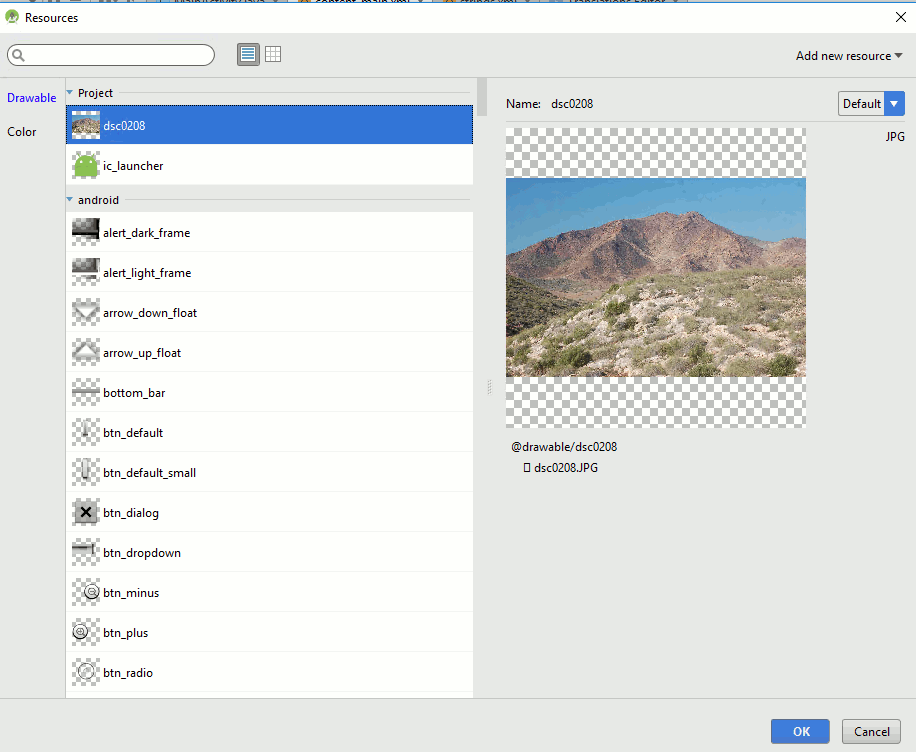
You will see that @drawable/desert has been entered as the value of the src property - you could have entered this string directly without the help of the Resources window.
You will also see the image displayed in the ImageView control:
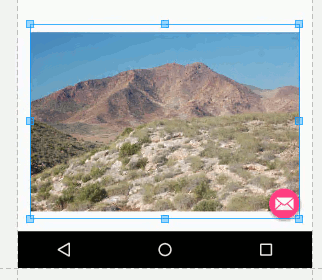
That's almost all there is to drawable/ resources, but there is a bit more to learn about the different types of drawables that you can store there. This is best discussed when we cover graphics in general in the next chapter.
Values
The easiest of the resource folders to use is probably values/, but it also tends to be the least often used.
The strings.xml file tends to be employed, but the others are underutilized. The reason for is is that the system tends to prompt you to enter strings as resources and there is an obvious advantage to string resources in that they allow easy localization. However putting constants of all types in resources is a good idea.
Although the main documentation only mentions string, arrays, colors, dimensions and styles, you can include a wider range of data types in the XML file:
Bool
<bool name="resourcename">true</bool>
Integer
<integer name="resourcename">1234</integer>
String
<string name="resourcename">A String</string>
There are also two array types:
Integer Array
<integer-array name="resourcename"> <item>123</item> <item>456</item> </integer-array>
Typeed Array
<array name="resourcename"> <item>resource</item> <item>resource</item>
</array>
Dimension and color are also two easy-to-understand value resources.
A dimension is simply an integer value complete with a units designator. For example:
<dimen name="resourcename">10px</dimen>
Obviously you can use any of the standard Android measurement scales - pt, mm, in and so on. You use dimensions anywhere that you need to specify a size or location in a particular set of units.
Color provides easy to use names for hex color codes. For example,
<color name="resourcename"> #f00 </color>
defines a red color.
You can specify a color using any of:
#RGB, #ARGB, #RRGGBB or #AARRGGBB
where each of the letters represents a single hex character with R= Red, G=Green, B=Blue and A=Alpha (transparency).
Ids
Ids are value resources and can be set up just like any other value resource. For example, if you want to set up an id for a button you might use:
<item type="id" name="resourcename" />
Notice that this is slightly different from over value resources in that you don't have to provide a value. The reason is that the system provides a unique integer value for each id.
You don't often need to define an id in an XML file all of its own because ids can be created on the fly within other XML files. Putting a + in front of the use of a resource id creates the resource without having to explicitly do the job.
For example
<Button android:text="@string/Greeting" android:id="+@id/button2"
creates the button2 resource but
<Button android:text="@string/Greeting" android:id="@id/button2"
will only work if you have defined button2 in a resource file in the values/ folder.
Of course in Android Studio you can simply type in an id in the property window say and the system will automatically provide the +@id to make it auto-create. Note that sometimes there are problems if you delete an id using Android Studio because it leaves +@id in the XML which isn't a valid id.
Accessing Resources In Code The R Object
For much of the time you really don't need to bother with accessing resources in code because the job is done for you automatically. For example if you assign a string resource to a button's text:
<Button
android:text="@string/Greeting"
Then the system retrieves the string resource and sets the button's text property to it when the layout is inflated. You don't have to do anything to make it all work.
However sometimes resources are needed within the code of an app and you have to explicitly retrieve them.
You already have some experience of retrieving resources because you have used R.id to retrieve id values but it is now time to find out exactly how this all works.
When your app is compiled by Android Studio it automatically creates a resource id - a unique integer - for every resource in your res/ directory. These are stored in a special generated class called R - for Resources. The directory structure in starting with res/ is used to generated properties for the R object that allows you to find the id that corresponds to any resource. This means that a resource id is always something like R.type.name. So for example
R.string.Greeting
Retrieves the resource id for the string resource with resource name "Greeting" i.e.
<string name="Greeting">Hello World</string>
Note that this is an integer resource id corresponding to Greeting - not its string value i.e. "Hello World".
So how do you convert a resource id to the resource value?
The first thing to say is that you don't always have to.
There are many methods that accept a resource id as a parameter and will access the resource on your behalf. It is usually important to distinguish when a method is happy with a resource id and when you have to pass it the actual resource value.
if you do have to pass the resource or you want to work with the resource then you have to make use of the Resources object. This has a range of gettype(resource_id) methods that you can use to access any resource.
For example to get the string with the resource name "Greeting" you would write:
Resources res= getResources();
String myString=res.getString(R.string.Greeting);
and myString would contain "Hello World". If you are not in the context of the activity you might have to use Context.getResources.
The only problem with using the Resources object is trying to work out which get method you actually need.
There are also methods that will return any part or all of its resource name give the id:
getResourceEntryName(int resid)
Return the entry name for a given resource identifier.
getResourceName(int resid)
Return the full name for a given resource identifier.
getResourcePackageName(int resid)
Return the package name for a given resource identifier.
getResourceTypeName(int resid)
Return the type name for a given resource identifier.
There are also some methods that will process the resource as well as simply retrieve it. For example, if you have raw XML resource getXml(int id) returns an XmlResourceParser that lets you work thought the XML retrieving tags, attributes, etc.
|