Page 1 of 3 So you know how to create an Android app, but do you really know how it works? Here's a basic guide in Kotlin, an extract from my published book Android Programming in Kotlin: Starting With An App.
Android Programming In Kotlin Starting with an App
Covers Android Studio 3 and Constraint Layout.
Is now available as a print book:
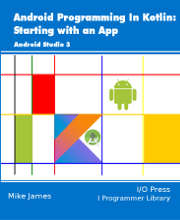
Buy from: Amazon
Contents
- Getting Started With Android Studio 3
- The Activity And The UI
Extract: Activity & UI
- Building The UI and a Calculator App
Extract: A First App
- Android Events
- Basic Controls
Extract Basic Controls Extract More Controls ***NEW!
- Layout Containers
Extract Layouts - LinearLayout
- The ConstraintLayout
Extract Bias & Chains
- Programming The UI
Extract Programming the UI Extract Layouts and Autonaming Components
- Menus & The Action Bar
- Menus, Context & Popup
- Resources
Extract Conditional Resources
- Beginning Bitmap Graphics
Extract Animation
- Staying Alive! Lifecycle & State
Extract State Managment
- Spinners
- Pickers
- ListView And Adapters
-
Android The Kotlin Way
If you are interested in creating custom template also see:
Custom Projects In Android Studio
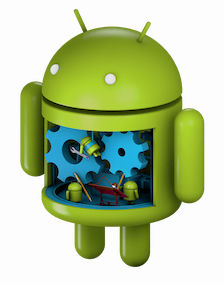
In this chapter we look at how to create a user interface (UI) and how to hook it up to the code in the Activity.
We discovered in Chapter 1 how to use Android Studio to build the simplest possible app. On the way we discovered that an Android app consists of two parts – an Activity and a View. The Activity is the code that does something and the View provides the user interface (UI). You can think of this duality as being similar to the HTML page and the JavaScript that runs to make it do something, or as a XAML form and the code behind.
The key idea is that an Activity is the code that works with a UI screen defined by the View. This isn't quite accurate in that an Activity can change its view so that one chunk of code can support a number of different views. However, there are advantages to using one Activity per view because, for example, this how the Android back button navigates your app – from Activity to Activity.
A complex app nearly always consists of multiple Activities that the user can move between like web pages but a simple app can manage quite well with just one Activity. There is no hard and fast rule as to how many Activities your app has to have, but it has to have least one.
If you are wondering if an Activity can exist without a View the answer is that it can, but it doesn't make much sense as this would leave the user with no way to interact with your app. Activities are active when their View is presented to the user.
It really is a great simplification to think in terms of an Activity as a single screen with a user interface.
If you want something to run without a UI then what you want is a service or a content provider which is beyond the scope of this book.
It is also worth making clear at this early stage that an Activity has only one thread of execution – the UI thread – and you need to be careful not to perform any long running task because this would block the UI and make your app seem to freeze. That is, an Activity can only do one thing at a time and this includes interacting with the user. If you write a program using a single activity and it does a complicated calculation when the user clicks a button then the activity will not be able to respond to any additional clicks or anything that happens in the UI until it finishes the calculation.
In most cases the whole purpose of the Activity that is associated with the UI is to look after the UI and this is what this book is mostly about.
Also notice that creating additional Activities doesn't create new threads. Only one Activity is active at any given time, more of this later when we consider the Activity lifecycle in detail.
In this book we are going to concentrate on the single screen UI Activity because it is the most common app building block you will encounter, and it is even where most complex apps start from.
The MainActivity
There is one activity in every project that is nominated as the one to be launched when your app starts. If you use Android Studio to create a new Basic Activity app called SimpleButton and accept all the defaults, the startup Activity is called MainActivity by default. You can change which Activity starts the app by changing a line in the app's manifest.
The Manifest is a project file we haven't discussed before because if you are using Android Studio you can mostly ignore it and allow Android Studio to construct and maintain it for you, but it is better if you know it exists and what it does.
The Manifest is stored in the app/manifests directory and is called AndroidManifest.xml.
It is an XML file that tells the Android system everything it needs to know about your app, including what permission it requires to run on a device.
In particular it lists all of the activities and which one is the one to use to start the app.
If you open the generated Manifest, by double-clicking on it, you will see a little way down the file:
<activity
android:name=".MainActivity"
android:label="SimpleButton" >
This defines the Activity the system has created for you and the lines just below this define it as the startup Activity:
<intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name= "android.intent.category.LAUNCHER" /> </intent-filter> </activity>
Notice that the choice of which Activity starts the app has nothing to do with what you call it, i.e. calling it MainActivity isn't enough.
For the moment you can rely on Android Studio to look after the Manifest for you. In most cases you will only need to edit it directly when you need to correct an error or add something advanced.
|