Page 1 of 3 The ESP32 S3 has WiFi, but getting from a simple connection to a web site is a matter of using the supplied client class. This is an extract from Harry Fairhead's latest book on programming the ESP32 using C and the Arduino library.
By Harry Fairhead
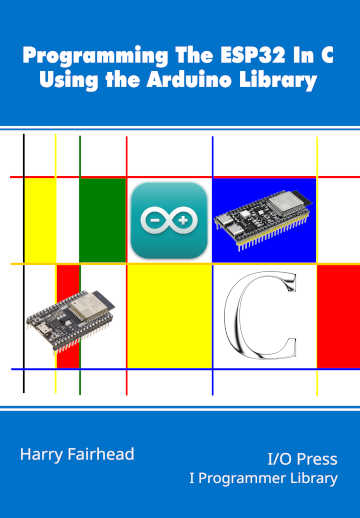
Available as a softback, hardback and kindle from Amazon
Contents
Preface
- The ESP32 – Before We Begin
- Getting Started
- Getting Started With The GPIO
- Simple Output
- Some Electronics
- Simple Input
- Advanced Input – Interrupts
- Pulse Width Modulation
Extract:PWM ***NEW!!!
- Controlling Motors And Servos
- Getting Started With The SPI Bus
- Using Analog Sensors
- Using The I2C Bus
- One-Wire Protocols
- The Serial Port
- Using WiFi
Extract: A Web Client
- Flash Files
- Direct To The Hardware
- Free RTOS
<ASIN:B0DHKT342W>
<ASIN:187196282X>
<ASIN:1871962919>
The ESP32 comes complete with a radio capable of 2.4GHz WiFi and Bluetooth. Most of the time you can ignore the technical details as there are easy-to-use functions which enable you to connect to a WiFi network and exchange data. In this chapter we look at the basics and how to create and use a WiFi connection. The libraries involved are many and extensive due to the need to cover a wide range of different protocols. A consequence is that there is no way to cover all of them in a reasonable space. This chapter is about getting started and understanding the basic structure of the WiFi and IP infrastructures. When you understand this the rest of the API becomes much easier to understand. The topic of Bluetooth is omitted as it is so varied that it deserves a book to itself.
In Chapter but not in this extract
- ESP32 Architecture
- The WiFi Stack
- IPAddress
- ESP WiFi
- ESP32 WiFi Events
A Practical Connect Function
Connecting to WiFi is a standard operation and it makes sense to package it in a function.
#include <WiFi.h>
int wifiConnect(char* ssid, char* password) {
int status = WiFi.begin(ssid, password);
while (status != WL_CONNECTED) {
switch (status) {
case WL_NO_SSID_AVAIL:
Serial.printf("No AP with name %s can be found", ssid);
return status;
case WL_CONNECT_FAILED:
Serial.printf("Connection failed");
return status;
case WL_CONNECTION_LOST:
Serial.printf("Connection lost possible security problem");
return status;
}
delay(100);
status = WiFi.status();
}
return status;
}
Using the functions connection becomes easy:
void setup() {
Serial.begin(9600);
int status=wifiConnect("ssid", "password");
Serial.println(status);
};
You can clearly customize the connection to include parameters to control the IP address, host name and authentication type. The delay in the while loop allows other tasks to run while waiting.
Alternatively you could use the ESP32 event system to make the connection asynchronous and to handle status changes after the connection:
#include <WiFi.h>
int status;
void WiFiEvent(WiFiEvent_t event) {
status = event;
Serial.println();
switch (event) {
case ARDUINO_EVENT_WIFI_READY:
Serial.println("WiFi interface ready");
break;
case ARDUINO_EVENT_WIFI_SCAN_DONE:
Serial.println("Completed scan for access points");
break;
case ARDUINO_EVENT_WIFI_STA_START:
Serial.println("WiFi client started");
break;
case ARDUINO_EVENT_WIFI_STA_STOP:
Serial.println("WiFi clients stopped");
break;
case ARDUINO_EVENT_WIFI_STA_CONNECTED:
Serial.println("Connected to access point");
break;
case ARDUINO_EVENT_WIFI_STA_DISCONNECTED:
Serial.println("Disconnected from WiFi access point");
break;
case ARDUINO_EVENT_WIFI_STA_GOT_IP:
Serial.print("Obtained IP address: ");
Serial.println(WiFi.localIP());
break;
case ARDUINO_EVENT_WIFI_STA_LOST_IP:
Serial.println("Lost IP address");
break;
default: break;
}
}
void wifiConnect(char* ssid, char* password) {
WiFi.onEvent(WiFiEvent);
status = WiFi.begin("ssid", "password");
}
Notice that the variable, status, is used to communicate with the rest of the program:
void setup() {
Serial.begin(9600);
wifiConnect("ssid", "password");
};
void loop() {
while (status != ARDUINO_EVENT_WIFI_STA_GOT_IP) {
delay(1000);
};
Serial.println(status);
delay(1000);
}
The event approach is more sophisticated and can detect additional states, but it is highly ESP-specific.
|