Page 1 of 3 Pins, GPIO Pins are the basis for all of IoT and in this very first extract from our latest book on using GPIO Zero on the Raspberry Pi in Python, we look at how to get started.
Raspberry Pi IoT In Python Using GPIO Zero Second Edition
By Harry Fairhead & Mike James
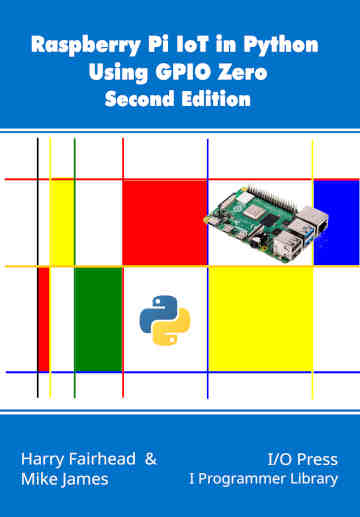
Buy from Amazon.
Contents
- Why Pi for IoT?
- Getting Started With Python And GPIO Zero
- Introduction to the GPIO
- Python - Class and Object
- Simple On/Off Devices
Extract 1: On/Off Devices *
- Pins And Pin Factories
Extract 1: Pins
- Some Electronics
- Simple Input
Extract 1:Getting Input ***NEW!!
- Complex Input Devices
Extract 1: Complex Input *
- Pulse Width Modulation
Extract 1: PWM*
- Controlling Motors And Servos
Extract 1: DC Motors *
- Working With Compound Devices
Extract 1: Compound Devices*
- The SPI Bus
- Custom SPI Devices
- Using The Lgpio Library
- Appendix Visual Studio Code Remote Python
*Extracts from first edition - will be updated.
<ASIN:1871962870>
Pins And Pin Factories
GPIO Zero is built on top of existing software libraries to the Pi’s hardware – to the GPIO lines hence GPIO libraries. You can use these libraries directly but they generally work in C and are not easy to get to grips with. To make things easier the GPIO libraries are wrapped by GPIO Zero Python classes, the pin factories. Their purpose is not only to create pin objects with the characteristics you specify, but to also manage the use of GPIO lines, ensuring that you cannot use the same GPIO line for two different purposes at the same time, and to provide a certain amount of event handling.
The reason pin factories are needed is that they cover up the differences between the different GPIO libraries and present a single uniform Pin object no matter which library you chose to use.
That is, in GPIO Zero a Pin object allows you to work directly with a GPIO line in the same way irrespective of the GPIO library in use.
As GPIO Zero selects a default pin factory you can mostly ignore the whole issue of pin factories and pins until you need to do something that goes outside of the box. So first let’s look at how to use a Pin object.
Raw GPIO Pin
In the Blinky program we used an LED object to turn the LED on and off – why not just reference the GPIO line? In other words, why hide the fact that we are working with GPIO lines? The answer is that it makes it easier to read programs. If you want to work with the raw GPIO line you can, but it isn’t as clear what the program means, i.e. what is the GPIO line controlling.
To use a pin from the pin factory that GPIO Zero has set up for you all you have to do is create a pin, set its function as 'input' or 'output' and then use it. For example, the pin equivalent of the blinking LED program is:
from gpiozero import Device
from time import sleep
Device()
pin=Device.pin_factory.pin(4)
pin._set_function('output')
while True:
pin.state=1
sleep(1)
pin.state=0
sleep(1)
Notice that you have to have a call to the Device constructor to initialize the pin_factory before you can use it. The pin factory creates a pin object for GPIO4 and then we use its methods to control what it does.
All pin objects have three fundamental methods:
_get_function
_set_function
_get_state
There is also a set of optional get/set methods which in practice all pin objects support. These methods are grouped together to implement properties:
Function
|
Input/Output
|
state
|
0/1
|
pull
|
Up/down/floating see next chapter
|
frequency
|
Frequency of output/none
|
bounce
|
Time in seconds/none, see Chapter 7
|
edge
|
Rising/falling/both/none, see Chapter 8
|
when_changed
|
Function called when an edge event occurs
|
There are also three general methods:
close
|
Releases pin and cleans up
|
output_with_state
|
Sets pin to output and given state
|
input_with_pull
|
Set pin to input with given pull, see Chapter 7
|
The output_with_state is useful because it sets the initial state of the GPIO line, i.e. where things start from. For example:
pin._set_function('output',1)
sets the pin to output and high.
You would probably agree that LED.on() is easier to understand than pin._set_state(1), but it isn’t a huge difference.
GPIO Zero uses the Pin class to build easier-to-use classes that implement the functionality of real devices like LEDs. Later this idea becomes even more useful as the Pin class is used to build more complicated devices that make use of multiple GPIO lines.
You may notice that the pin’s methods start with an underscore which is the usual way of indicating that a method is private and should not be used. This is because the Pin class is intended to be used as the basis for other classes like LED and so on, rather than being used directly. Later we will discover how to use it to create new, higher-level, classes similar to LED. That is, if you want to add a new device to GPIO Zero then at first you might use the Pin class to get a prototype working, but after this stage you should rewrite the code so that the pins are hidden and the object works in more natural terms. For example, if you have a new gas sensor you might first use pin objects to get it working, but the final Gas object would have methods like get_reading, calibrate and so on with no mention of GPIO lines at all.
|