Page 1 of 4 JavaScript is an event driven language - which means you can't avoid dealing with events. jQuery doesn't just provide a browse-independent way of working with events, it reinvents the event system. With jQuery you really can write less and do more when it comes to events.
Just jQuery Events, Async & AJAX
Is now available as a print book: Amazon
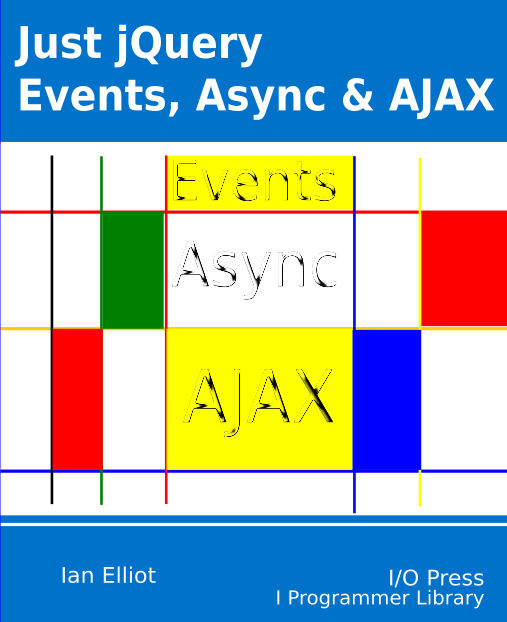
Contents
- Events, Async & Ajax (Book Only)
- Reinventing Events
- Working With Events
- Asynchronous Code
- Consuming Promises
- Using Promises
- WebWorkers
- Ajax the Basics - get
- Ajax the Basics - post
- Ajax - Advanced Ajax To The Server
- Ajax - Advanced Ajax To The Client
- Ajax - Advanced Ajax Transports And JSONP
- Ajax - Advanced Ajax The jsXHR Object
- Ajax - Advanced Ajax Character Coding And Encoding
Also Available:
buy from Amazon
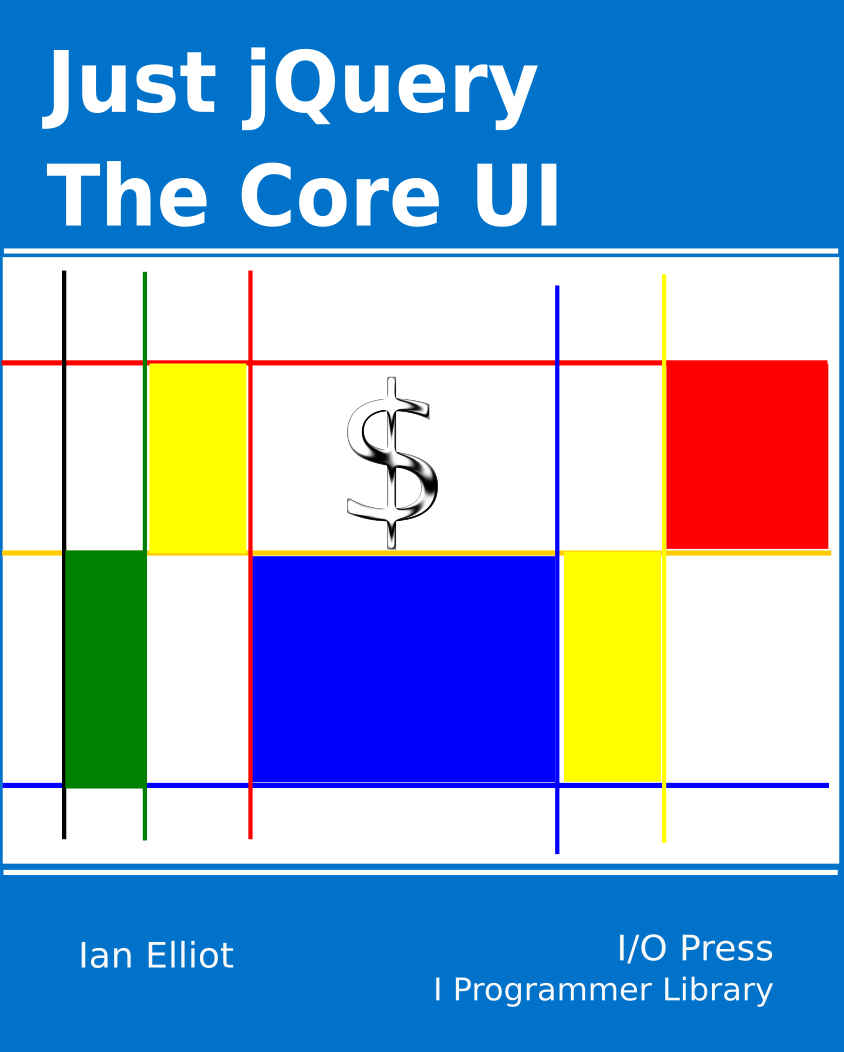
Advanced Attributes
Events are central to JavaScript and to all JavaScript programs. You simply cannot afford to not fully understand them. In this chapter we introduce the idea of an event and an event handler and show how jQuery provides lots of help for you to make use of events and even extends the way events work.
First let's find out about events work in pure JavaScript.
Events
An event is something that happens at a particular time and at a particular location.
For example, the user might click a button or any element in the UI. Notice that an event is associated with a particular element. There isn't a single click event, but a click event can occur for every element in the UI that the user can click on.
You can associate a function, an event handler, with any event and the function will be called when the event occurs. This gives you the basic structure of a JavaScript program which is essentially a collection of event handlers.
The important thing to realize is that JavaScript is single-threaded. That is, there is only one thread of execution in a JavaScript program. This means that there is only one instruction being executed at any moment. But events can occur at any time so how do we cope with an event that occurs when the program is already doing something else?
What happens is that there is an event or dispatch queue to which the event is added until it can be dealt with. The system examines the dispatch queue and if there is an event waiting to be processed it takes it off the queue and calls the appropriate event handler. The event handler then runs to completion and only then does the system go back to the dispatch queue to see if there is another event to process.
Also notice that an event is only added to the dispatch queue if there is an event handler associated with it. Events that don't have handlers are simply thrown away.
The JavaScript Way
The fact that JavaScript is single-threaded makes it very easy to reason about programs because two or more things cannot happen at any one time. In JavaScript only one thing is happening at any given time.
However it also means that if you write an event handler that takes a long time to complete then nothing else can happen. To be specific while an event handler is running no other event can be processed - they simply join the event queue - and the user is presented with a program that seems to be frozen.
A JavaScript program is a collection of event handlers waiting for something to happen.
The ideal JavaScript program doesn't do anything at all so allowing the system to wait for an event to enter the dispatch queue. This allows the system to respond to any user actions at once. The event handler that responds also does zero work and lets the system get back to waiting for the next event as quickly as possible. Anything that the program does simply slows the response to the user interface.
Of course this isn't possible because all programs actually have to do something to be useful. What you have to do is to keep in mind at all times that while your event handler is running the UI is frozen. Keep event handlers short, and if you can't you have to find other ways of either breaking up the computation or shifting it to another thread. It is also important to understand that it isn't so much the total amount of work that has to be minimized. What really matters is how often you yield control back to the system. You can get a lot of work done if you break it down into 50 millisecond chunks, for example.
These are topics discussed in later chapters.
HTML Events
Now that we know how events and the dispatch queue work, it is time to look at how HMTL events are implemented using just JavaScript - jQuery's improved event handling will be easier to appreciate when you know what it is replacing.
In HTML you can set an event handler on an element using the well-known "on" attributes - e.g.
<button onclick="myEventHandler" ...
In code the modern way of doing the same job is to use the addEventListener method to register the event handler to the target.
For example:
button1.addEventListener("click",myEventHandler);
will result in myEventHandler being called when the user clicks the button.
Notice that while the HTML needs a string which provides the event handler's name, the addEventListener uses the reference to the function object. The first parameter specifies the event as a string. You can look up the names of all of the events in the documentation.
One small point that is often overlooked is that an event source can have more than one event handler registered with it.
For example,
button1.addEventListener("click",myEventHandler1); button1.addEventListener("click",myEventHandler2);
function myEventHandler1(e){ alert("event1"); }
function myEventHandler2(e){ alert("event2"); }
when the button is clicked you will see two alerts, one per event handler. Notice that there is no guarantee as to the order in which the event handlers are called.
Why would you register multiple event handlers?
In many cases it doesn't make sense to use more than one event handler. The reason is that an event occurs and it is clear and simple what should happen. The user clicks a Save button and the click event should result in a click event handler being called that saves the data.
One event - one handler.
However, there are times when this isn't the case because an event is associated with multiple unrelated logical actions.
You might have a Save button that saves the data and incidentally shows the user an ad. In this case it makes sense to keep the functions separate. However, notice that there is no way to make sure that the ad is shown before the save or vice versa.
You can also remove an event handler using:
removeEventListener(type,function)
Notice that the type and function have to match exactly.
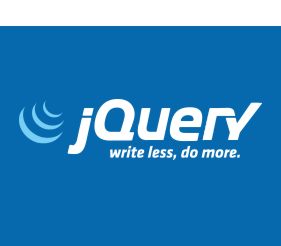
|