Page 1 of 2 JavaScript's way of working with dates is simple but perhaps this is part of the problem. The Date object is so simple that it can be difficult to work out how to do things like date arithmetic. Find out how to get up to speed with dating ...
JavaScript Data Structures
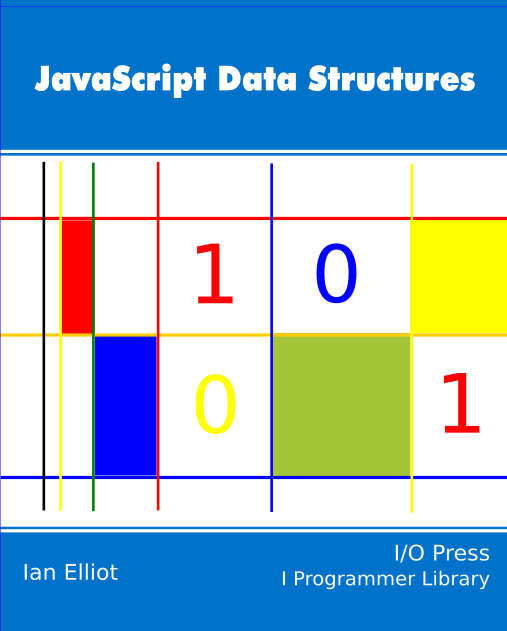
Contents
- The Associative Array
- The String Object
- The Array object
- Speed dating - the art of the JavaScript Date object
- Doing JavaScript Date Calculations
- A Time Interval Object
- Collection Object
- Stacks, Queue & Deque
- The Linked List
- A Lisp-like list
- The Binary Tree
- Bit manipulation
- Typed Arrays I
- Typed Arrays II
- Master JavaScript Regular Expressions
* First Draft
Have you ever tried using JavaScript dates?
They are simple enough in theory but in practice things can get complicated. In particular there is the getTime method which returns the date/time as a numeric value - sometimes you seem to need it and other times (no pun intended) you don't.
Then there is the way you sometimes have to create a new date object to hold the result of a date and time computation and sometimes you don't. For the beginner, or for the occasional date user, it can be confusing.
Dates in JavaScript are easy to make use of once you understand how they are implemented and once you realize how the JavaScript's type juggling gets into the act.
First some Date basics.
More than the age of the universe
The key idea in using a Date object is to know that it stores the date and time as the number of milliseconds from the fixed date:
Thursday Jan 1st 00:00:00 UTC 1970.
Why this exact date in 1970?
The reason is that this is the Unix epoch, i.e it is around the time when the Unix operating system was created. Keeping time in seconds from this date is usually called "Unix time". Notice that JavaScript uses the Unix epoch but it counts time in milliseconds ticks, not in seconds. That is:
JavaScript time (millisecond ticks) = Unix time*1000
There is also the complication that JavaScript doesn't store its times as simple 32-bit integers but as integers represented as 64-bit floating point values. This gives you an exact integer range of plus or minus 253 which is 9,007,199,254,740,992
However the Date object doesn't allow you to use this full range. It restricts the number of millisecond ticks to be in the range plus or minus:
8,640,000,000,000,000
which gives you a date range of plus or minus
100,000,000,000,000 days.
To put this another way you can work with dates that span more than 23 billion (109) years before and after 1970. This should be enough for most, as the age of the universe is estimated to be around 13 billion years.
Notice that positive values are used to indicate dates after 1970 and negative values are before the fixed date.
Static methods
The Date object is derived fairly directly from the Function object but it has lots of extra methods and properties. The Date object is a constructor function and it has lots of prototype methods defined for dealing with dates and times.
It also has some additional methods which behave like static or class methods. In JavaScript it is the general rule that additional methods of the constructor function object behave like class methods. These static methods are supported by all modern browsers but not by older versions- IE 8 for example - however, they are easy to add.
The most useful of the static methods is now, which simply returns the number of milliseconds from the fixed date to the current moment. That is:
var t=Date.now();
stores the current time and date in t.
Notice that t isn't a Date object. just a numerical value. If you use:
alert(t);
then you will see a large number not a date.
The other two slightly less useful static methods are:
Date.UTC(year,month,day,hour,minute, second,millisecond);
which returns the number of milliseconds since the fixed date for the specified date and time (all of the time parameters are optional) and
Date.parse(string);
which parses the date in the string and returns the number of milliseconds.
Notice that all of these static functions return simple numeric values and not a date object.
Also notice that earlier versions of JavaScript didn't support these static methods.
The date instance
The next level of sophistication is to create a Date instance.
The Date function object is the constructor for any instances of the Date object you care to create. There are four variations on the way the constructor can be called:
new Date();
returns a Date object with the current date and time
new Date(milliseconds);
returns a Date object with number of milliseconds from the fixed date
new Date(dateString);
returns a Date object set to the specified date and time as specified by the date string
and finally
new Date(year,month,day,hour,minute,
second,millisecond);
returns a Date object set to the specified date and time.
The only complication is that you need to remember that in the constructor the first month of the year is month zero not month one. So
new Date(1970,1,1);
isn't the first of January but of February.
Notice that you really do need to think about the Date object as storing the number of milliseconds from the fixed date rather than as storing a date and a time in some other format.
That is:
- a Date object stores a number corresponding to the number of milliseconds since the fixed date and you can think of this as its value.
You can always discover the number of milliseconds stored in the Date object using the getTime method and you can set it using the setTime method.
UTC and local time
There are lots of methods that will use the value of a Date object to return other information in other data formats.
The most basic are the UTC functions which return the date and time as stored in the Date object.
- get/setUTCDate the day (date) of the month (1-31)
- get/setUTCDay the day of the week (0-6)
- get/settUTCFullYear the year (4 digits for 4-digit years)
- get/setUTCHours the hours (0-23)
- get/setUTCMilliseconds the milliseconds (0-999)
- get/setUTCMinutes the minutes (0-59)
- get/setUTCSeconds the seconds (0-59)
Of course not everyone wants to work with UTC and there are local time equivalents of each of the above methods - just leave out the UTC part of the name. Local time is offset from UTC by a number of minutes. If you use one of these local time methods to set a Date object then the time is first converted to UTC and then the number of UTC millisecond ticks is computed and stored in the Date object. Similarly when you get a local time the UTC time is converted to local time.
The rule is that the Date object always stores the UTC time and conversions to and from local time are performed unless you use the UTC methods.
You can discover the local time offset using the getTimezoneOffset function which returns the time-zone offset in minutes for the current locale.
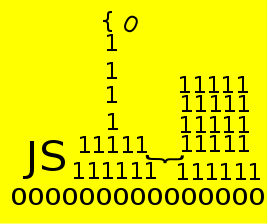
<ASIN:0596517742> <ASIN:0596805527> <ASIN:0470684143>
|