Page 1 of 2 The whole of the JavaScript language is built on one central data structure - the associative array. Objects in JavaScript are just associative arrays and this causes a lot of confusion at first. But the fact of the matter is that the associative array is used to build every other type of data structure in JavaScript.
JavaScript Data Structures
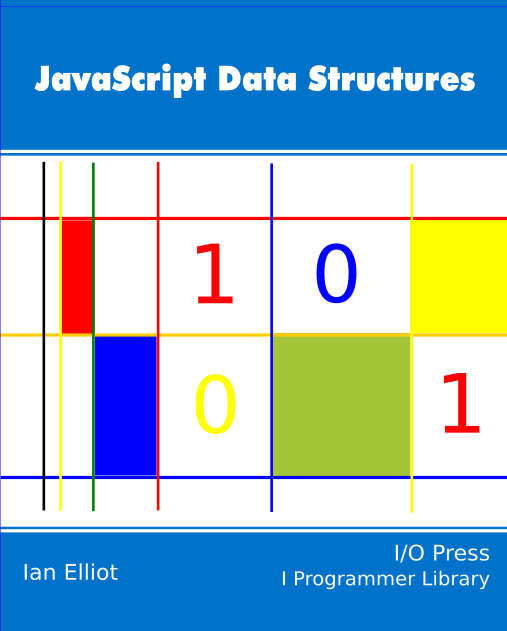
Contents
- The Associative Array
- The String Object
- The Array object
- Speed dating - the art of the JavaScript Date object
- Doing JavaScript Date Calculations
- A Time Interval Object
- Collection Object
- Stacks, Queue & Deque
- The Linked List
- A Lisp-like list
- The Binary Tree
- Bit manipulation
- Typed Arrays I
- Typed Arrays II
- Master JavaScript Regular Expressions
* First Draft
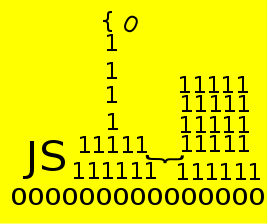
The whole of the JavaScript language is built on one central data structure - the associative array. It is usual to say that everything in JavaScript is an object but it might be more accurate to say that everything in JavaScript is an associative array. The reason for this is that a JavaScript object is just an associative array that stores other JavaScript objects which are, of course associative arrays.
What this means is that if you want to create other data structures in JavaScript you have to start with an associative array.
The Associative Array
Many JavaScript programmers get very confused about the way that the Array object works. It seems to be some sort of advanced form of the familiar numerically indexed array. Before we look at the Array object itself the associative array deserves consideration in its own right.
As already stated the key idea is that every Javascript object is an associative array which is the most general sort of array you can invent - sometimes this is called a hash or map structure or a dictionary object.
An associative array is simply a set of key value pairs.
The value is stored in association with its key and if you provide the key the array will return the value.
This is all an associative array is and the name comes from the association between the key and the value. The key is a sort of generalized address that can be used to retrieve the stored value.
For example:
array={key1: 'value1',key2:'value2'};
creates an object called array with two keys and two values which in this case happen to be two constant strings.
Notice that the value stored can be any JavaScript object and in this example it is probably better to think of storing two string objects rather two string literals.
The key can be either an identifier, a string or a number but more about the difference in the key type as we progress.
You can retrieve a value via it key using array notation:
alert(array['key2']);
Which displays the string value2. If you try and access a key that doesn't exist then you get the result undefined.
Notice that there is something slightly odd in this syntax in that when you create the associative array the keys were entered as if they were names (of variables say) rather then strings. However when you access an array the key has to be specified as a string.
The reason for this is that the array notation allows you to use an expression as in:
alert(array['key'+2]);
and because of this the key specification has to evaluate to a string. Notice that by the very relaxed rules of JavaScript data typing 'key'+2 is interpreted as 'key'+'2' which evaluates to the string 'key2'.
In short it doesn't matter if you specify the key as an identifier or a string it all works as if you had specified a string. In fact if you want to you can always specify the key as a string e.g.
array={'key1': 'value1','key2':'value2'};
It helps to think of this in terms of the square brackets [] as being the array operator that is
object[string]
evaluates to the value associated with the key specified by string stored in the associative array object.
You can change the value associated with a key simply by using the array notation to assign the new value, e.g.
array['key2']='new value';
You can also add a key/value pair at any time simply by using the array notation to access a key that doesn't exist:
array['key3']='value3';
As the associative array is used to as the basis of the JavaScript object there is an alternative way to access a value that makes the key look like a property. That is you can access the value using "property syntax" as in:
alert(array.key2);
You can also change the value using assignment to a property
array.key2='new value';
and you can add a new key/value pair by assigning to a property that doesn't exist:
array.key3='value3';
Notice that what you can't do with property notation is specify an expression as the key. That is you cannot write:
array.key+'2"='new value';
When you specify a key using property notation it has to be fully determined at compile time. This means that array notation is more powerful than property notation.
It is the availability of property notation that means that we can treat a JavaScript associative array as an object.
In ECMAScript 2015 you can also use expressions in keys as part of array definitions:
array={'key1': 'value1',['key'+2]:'value2'};
The second key evaluates to 'key2'. Notice the use of the array [] operator and also notice that this doesn't work in earlier versions of JavaScript.
Now that you have encountered the JavaScript associative array it is worth restating that there is no other data structure in JavaScript. The amazing thing is that everything else in JavaScript is constructed from this one powerful data structure.
Associative arrays as objects
Notice that the object/property syntax is just that - some alternative syntax for accessing an associative array. It is quite a thought that all of JavaScript's object- oriented features come from the associative array plus one additional operator.
You can already store any object in an associative array so you have the equivalent of object properties. All that seems to be missing are object methods but they too are included.
JavaScript has a special type of object called a function object which has a special characteristic that you can associate code with it. That is in JavaScript a function is just an object that stores some executable code.
The function evaluation operator () will cause any function object to execute its code. It will also initialize any parameter values and it evaluates to the return value of the function. As a function object is just a standard JavaScript object it can be stored in an associative array as a value - this is why an associative array can be used as objects.
For example:
array={'key1':'value1', 'key2':function(){alert("HelloMethod");}};
The object associated with key1 is just a string object and the object associated with key2 is a function object and you can retrieve it in the usual way
var method=array['key2'];
and if you display the variable method's contents
alert(method);
you will discover that it is the text of the function:
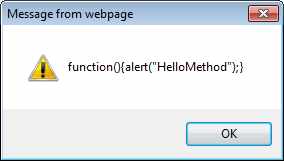
Don't be fooled by this, the method variable doesn't contain a string; it is a function object as you can prove by:
alert(typeof(method));
which displays:
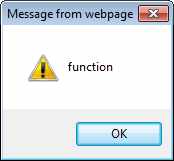
<ASIN:1871962579>
<ASIN:1871962560>
<ASIN:1491901888>
<ASIN:144934013X>
<ASIN:193398869X>
<ASIN:1449373216>
|