Page 1 of 2 There are many ways in which JavaScript's approach is different from standard, run of the mill, class-based languages, but some of them go unnoticed. Take the whole subject of parameter passing - JavaScript does it different.
This is an extract from the book Just JavaScript by Ian Elliot.
Buy Now: from your local Amazon
Just JavaScript An Idiomatic Approach
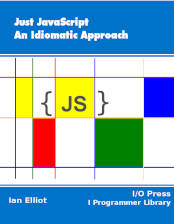
A Radical Look At JavaScript
Most books on JavaScript either compare it to the better known class based languages such as Java or C++ and even go on to show you how to make it look like the one of these.
Just JavaScript is an experiment in telling JavaScript's story "just as it is" without trying to apologise for its lack of class or some other feature. The broad features of the story are very clear but some of the small details may need working out along the way - hence the use of the term "experiment". Read on, but don't assume that you are just reading an account of Java, C++ or C# translated to JavaScript - you need to think about things in a new way.
Just JavaScript is a radical look at the language without apologies.
Contents
- JavaScript – Essentially Different
- In The Beginning Was The Object
- Real World Objects
- The Function Object
Extract - The Function Object Extract - Function Object Self Reference
- The Object Expression
- Function Scope, Lifetime & Closure
Extract Scope, Lifetime & Closure Extract Execution Context ***NEW!
- Parameters, Returns and Destructuring
Extract - Parameters, and Destructuring
- How Functions Become Methods
- Object Construction
Extract: - Object Factories
- The Prototype
Extract - ES2015 Class and Extends
- Inheritance and Type
- The Search For Type
- Property Checking
Buy Now: from your local Amazon
Also by Ian Elliot JavaScript Async: Events, Callbacks, Promises and Async Await Just jQuery: The Core UI Just jQuery: Events, Async & AJAX
<ASIN:1871962579>
<ASIN:1871962560>
<ASIN:1871962501>
<ASIN:1871962528>
In this short chapter we look at some of the more practical aspects of using Functions in JavaScript. There is nothing deep and philosophical about the ways that we can use JavaScript’s functions but it does give you some idea of how powerful the idea that “every thing is an object” and the weak typing are.
Object Parameters
Another big difference between JavaScript and most other languages is that parameters in functions and return values can be objects of any type – in fact it is easier to forget ideas of type and simply regard parameters as objects.
This has the effect of also removing any ideas of function signature i.e. the types of the parameters and hence of function overloading. If you are familiar with languages where both of these ideas are central to object oriented programming this will seem to be a big loss however what you have in return is the idea that a function can be written to deal with any objects that happen to be passed to it.
For example in other languages you might well have to write a function to add two integers and another to add two floats. If you want a function that works with both integers and floats then you have to invent generics and deal all of the complications that this brings. In JavaScript you can simply write:
function add(a,b){ return a+b;
}
and use the function to add two integers or two decimal valued numbers or even two strings although in this case the result is concatenation rather than addition. What is more you can pass any two objects to the add function without generating any compile time error. For example:
alert(add({},{}));
There are no restrictions on what sort of object you can pass to a function as a parameter and no restriction on what the function can return.
This freedom can seem like an invitation to chaos but in there are many simplifications that also result. For example, there is no need to event the complex subject of generics because all JavaScript functions are generic.
The whole idea of typeless programming is taken up in later chapters but this is where it first makes itself felt.
Arguments Object
The fact that parameters are objects is a powerful feature but, as mentioned in an earlier chapter, in fact the entire parameter list is an object.
Every function, apart from arrow functions, has a local arguments object which contains all of the parameter values passed to the function in something that looks like an Array. What is more the function declaration does not determine what can be passed to the function at the point of use.
That is function declarations do not need to specify any parameters at all and you can call any function with any list of arguments you care to use.
For example:
function sum(){
var total=0; for(var i=0;i<arguments.length;i++){ total+=arguments[i];
} return total;
}
which will add any number of parameters you care to specify. Notice that the declaration does not specify anything at all, not event their number, about the parameters that the function expects. You can call it using:
alert(sum(1,2,3,4));
or with any number of parameters of any type.
The arguments list is processed by the JavaScript engine to produce an object that is like an Array.
This is really all we need to write any function and indeed other languages struggle to provide this level of flexibility.
So why do we have a parameter list in function declarations?
The simple answer is to make JavaScript functions look more like functions in other languages and to make simple functions easier to write. Any parameter list that you specify in the declaration is used to deconstruct the arguments object into named local variables.
For example:
function sum(a,b,c){
}
unpacks the first three parameters you pass into a, b and c respectively. If you pass fewer than three then any parameters that do not have values are undefined. If you pass more than three then only the first three values are used in the local variables but of course all of the values are still available in the arguments object.
This unpacking or destructuring of the arguments object really is a good way to think about how parameters work in JavaScript. For example there is a two way connection between the parameter variables and the arguments object. If you change the arguments object then the value in the variable changes and vice versa.
For example:
function myFunction(a){ alert(a); arguments[0]=1; alert(a);
a=2; alert(arguments[0]); }
MyFunction(0);
displays 0, 1 2 and changing arguments changes the associated parameters and vice versa.
Strict Mode
The association between arguments and parameters is broken in strict mode. Any changes to arguments do not change the parameters and vice versa.
Passing An Array To arguments - Spread
If parameters are passed to functions in the arguments array you might be thinking if you can bypass the comma separated list of parameters and simply pass an Array that is used as arguments.?
You can but only if you call the array using either the apply method that every Function object has or you are prepared to use some new syntax introduced with ES2015.
First to pass a parameter array in almost any version of JavaScript you can use apply:
function sum(a,b){ return a+b; };
var args=[1,2];
sum.apply(null,args);
This passes the args array to the function as the arguments object which is then unpacked into the usual parameters.
Using the ES2015 spread operator … you can write the above as:
function sum(a,b){ return a+b; };
var args=[1,2]; sum(...args);
The spread operator will unbundle any iterable object like an array and it works in function arguments, elements of array literals and object expressions where key value pairs are needed.
|